Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial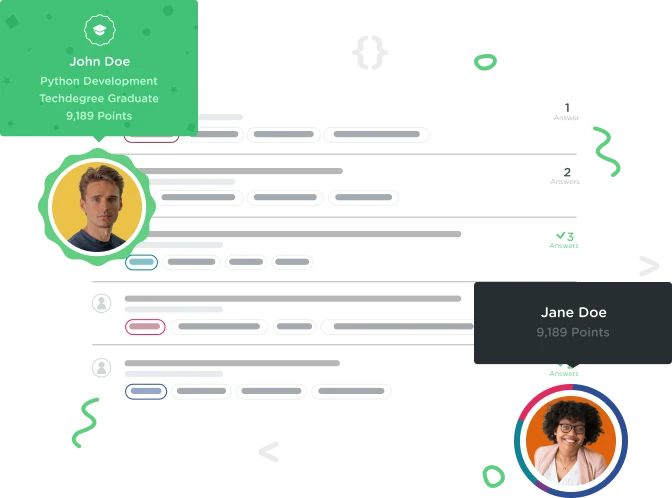

Juan Manuel Ahumada
4,056 PointsError: "undefined method `todo_items' for #<TodoList:0xba229fc4>" when running rspec and when displaying todo_item view
I'm receiving that error just after I added the following code in todo_items view (app/views/todo_items/index.html.erb)
<ul class="todo_items"> <% @todo_list.todo_items.each do |todo_item| %> <li><%= todo_item.content %> </li> <% end %> </ul>
At this time, my Models look like this: app/models/todo_item.rb class TodoItem < ActiveRecord::Base belongs_to :todo_list end
app/models/todo_list.rb class TodoList < ActiveRecord::Base has_many :todo_item validates :title, presence: true validates :title, length: {minimum: 3} validates :description, presence: true validates :title, length: {minimum: 5} end
My Controllers: app/controllers/todo_items_controller.rb class TodoItemsController < ApplicationController def index @todo_list = TodoList.find(params[:todo_list_id]) end end
app/controllers/todo_lists_controller.rb class TodoListsController < ApplicationController before_action :set_todo_list, only: [:show, :edit, :update, :destroy]
# GET /todo_lists # GET /todo_lists.json def index @todo_lists = TodoList.all end
# GET /todo_lists/1 # GET /todo_lists/1.json def show end
# GET /todo_lists/new def new @todo_list = TodoList.new end
# GET /todo_lists/1/edit def edit end
# POST /todo_lists # POST /todo_lists.json def create @todo_list = TodoList.new(todo_list_params)
respond_to do |format|
if @todo_list.save
format.html { redirect_to @todo_list, notice: 'Todo list was successfully created.' }
format.json { render action: 'show', status: :created, location: @todo_list }
else
format.html { render action: 'new' }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /todo_lists/1 # PATCH/PUT /todo_lists/1.json def update respond_to do |format| if @todo_list.update(todo_list_params) format.html { redirect_to @todo_list, notice: 'Todo list was successfully updated.' } format.json { head :no_content } else format.html { render action: 'edit' } format.json { render json: @todo_list.errors, status: :unprocessable_entity } end end end
# DELETE /todo_lists/1 # DELETE /todo_lists/1.json def destroy @todo_list.destroy respond_to do |format| format.html { redirect_to todo_lists_url } format.json { head :no_content } end end
private # Use callbacks to share common setup or constraints between actions. def set_todo_list @todo_list = TodoList.find(params[:id]) end
# Never trust parameters from the scary internet, only allow the white list through.
def todo_list_params
params.require(:todo_list).permit(:title, :description)
end
end
1 Answer
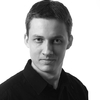
Maciej Czuchnowski
36,441 Pointshas_many :todo_item
should be
has_many :todo_items
Juan Manuel Ahumada
4,056 PointsJuan Manuel Ahumada
4,056 PointsThank you, with that change, the issue was solved.