Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial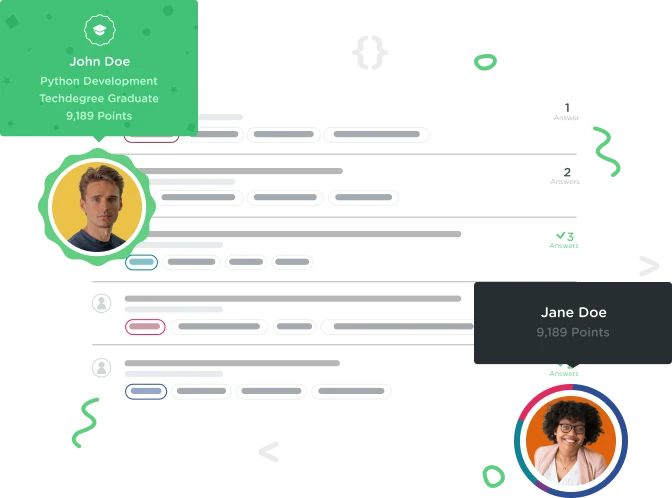

Anthony Renwick
3,609 Pointserror: unreported exception IOException; must be caught or declared to be thrown - String story = prompter.newPrompt();
Hello everybody, I have an issue that seems to be getting in the way of completing my assignment in Finishing TreeStory.
I tried to add catch to newPrompt but that didn't work. And even when I add in the 'throws IOException' in newPrompt, I get additional errors. Thankfully, I'm getting close to taking care of this assignment, but I need that push in the right direction.
Can anyone give me that push?
Code (Main.java):
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = prompter.newPrompt();
Template tmpl = new Template(story);
try {
prompter.promptForWord(story);
} catch (IOException e) {
e.printStackTrace();
}
prompter.run(tmpl);
}
}
Code (Prompter.java):
package com.teamtreehouse;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Scanner;
import java.util.Set;
public class Prompter {
private final Set<String> mCensoredWords;
public Prompter() {
mCensoredWords = loadCensoredWords();
}
private static Set<String> loadCensoredWords() throws IOException {
Path file = Paths.get("resources", "censored_words.txt");
return new HashSet<>(Files.readAllLines(file));
}
public void run(Template tmpl) {
List<String> results = promptForWords(tmpl);
String res = tmpl.render(results);
System.out.format("Your TreeStory: %s", res);
}
public String newPrompt() throws IOException {
try (Scanner scan = new Scanner(System.in)) {
System.out.print("Enter a prompt: ");
return scan.nextLine();
}
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
try (Scanner scan = new Scanner(System.in)) {
while (true) {
System.out.format("Enter a word that is a(n) %s%n: ", phrase);
String userInput = scan.nextLine();
if (mCensoredWords.contains(userInput))
System.out.println("Try a less offensive word");
else
return userInput;
}
}
}
}
Error Message:
./com/teamtreehouse/Main.java:10: error: unreported exception IOException; must be caught or declared to be thrown
String story = prompter.newPrompt();
^
1 error
2 Answers
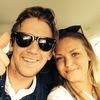
Jonathan Hermansen
25,935 PointsLooks like your readLine variable on line 76 are empty (e.g. null), therefore you get NullPointerException. You should check if the new BufferedReader(new InputStreamReader(System.in)) in your contructor actually pass data to the mReader variable.
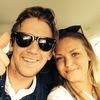
Jonathan Hermansen
25,935 PointsWhen using the throws keyword, be sure to also throw new exception. When using the catch-block, be sure to catch an exception that may be thrown in the try-block, e.g. catch(TypeOfException ex) { ... }.
You could for example start with the following:
Main.java
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = prompter.newPrompt();
Template tmpl = new Template(story);
try {
prompter.promptForWord(story);
} catch (Exception ex) { // Use specific exception instead of general 'Exception' that may be thrown in try-block
// error message
}
prompter.run(tmpl);
}
}
Prompter.java
package com.teamtreehouse;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Scanner;
import java.util.Set;
import java.util.*;
public class Prompter {
private final Set<String> mCensoredWords;
public Prompter() {
mCensoredWords = loadCensoredWords();
}
private static Set<String> loadCensoredWords() {
try {
Path file = Paths.get("resources", "censored_words.txt");
return new HashSet<>(Files.readAllLines(file));
} catch (Exception ex) { // Use specific exception instead of general 'Exception' that may be thrown in try-block
// error message
}
return Collections.emptySet();
}
public void run(Template tmpl) {
List<String> results = promptForWords(tmpl);
String res = tmpl.render(results);
System.out.format("Your TreeStory: %s", res);
}
public String newPrompt() {
String input = "";
try (Scanner scan = new Scanner(System.in)) { // Use specific exception instead of general 'Exception' that may be thrown in try-block
System.out.print("Enter a prompt: ");
input = scan.nextLine();
return input;
} catch (Exception ex) {
// error message
}
return input;
}
public List<String> promptForWords(Template tmpl) {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) {
String userInput = "";
try (Scanner scan = new Scanner(System.in)) {
while (true) {
System.out.format("Enter a word that is a(n) %s%n: ", phrase);
userInput = scan.nextLine();
if (mCensoredWords.contains(userInput))
System.out.println("Try a less offensive word");
else
return userInput;
}
} catch (Exception ex) { // Use specific exception instead of general 'Exception' that may be thrown in try-block
// error message
}
return userInput;
}
}
This would work, but you should catch more specific exceptions, the Exception.java is a general exception class that other more specific exceptions inherit from. So when debugging an application, the general Exception does not tell you specific what went wrong, compared to an InvalidPathException, when you for example use Paths.get("resources", "censored_words.txt"). Check the documentation for the classes and methods you use to see what exceptions they may throw (under specific method, and under Throws-row)
You should also only have code that may throw exceptions inside the try-catch-blocks.

Anthony Renwick
3,609 PointsHey Jonathan,
I did as you said but, I am getting new errors now. Even when I looked in the Forum to see how these errors were resolved and applied different solutions, I have had no such luck as other errors came about. But for now, I will share what the main errors are that I am dealing with:
Error:
java.lang.NullPointerException
at com.teamtreehouse.Prompter.promptForWord(Prompter.java:81)
at com.teamtreehouse.Prompter.promptForWords(Prompter.java:65)
at com.teamtreehouse.Prompter.run(Prompter.java:42)
at com.teamtreehouse.Main.main(Main.java:20)
at JavaTester.run(JavaTester.java:77)
at JavaTester.main(JavaTester.java:39)
Main.java Code:
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = prompter.newPrompt();
Template tmpl = new Template(story);
try {
prompter.promptForWord(story);
} catch (Exception ex) {
System.out.println("Could not load prompt");
ex.printStackTrace();
}
try {
prompter.run(tmpl);
} catch (Exception ex) {
System.out.println("Could not run prompt");
ex.printStackTrace();
}
}
}
Prompter.java Code:
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException ex) {
System.out.println("Could not load censored words");
ex.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (Exception ex) {
System.out.println("There was a problem");
ex.printStackTrace();
System.exit(0);
}
String render = tmpl.render(results);
System.out.printf("Your TreeStory: %n%n%s", render);
}
public String newPrompt() {
String readLine = "";
try {
readLine = mReader.readLine();
} catch (IOException ex) {
System.out.printf("Problem getting input. %s\n", ex.getMessage());
}
return readLine;
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) {
String readLine = "";
try {
readLine = mReader.readLine();
} catch (Exception ex) {
System.out.printf("Problem getting input. %s\n", ex.getMessage());
}
for (String censoredWord : mCensoredWords){
if (readLine.equals(censoredWord)){
System.out.printf("Try a less offensive word. \n");
readLine = promptForWord(phrase);
}
}
return readLine;
}
}
Anthony Renwick
3,609 PointsAnthony Renwick
3,609 PointsFirst off, thank you for the answer. I am also running into another problem. I looked through the Forum to see where I was going wrong, and I was not able to get past the error. If I may ask, do I need to add another line in promptForWords, or something like that?
Prompter.java:
Error Message: