Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial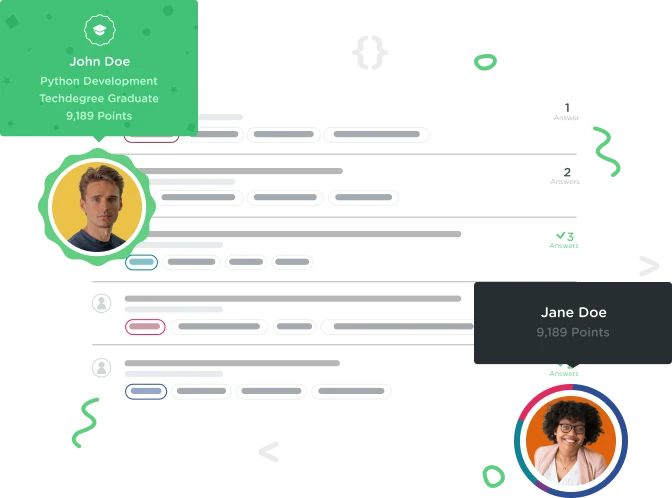

Chanda Lupambo
Courses Plus Student 27,336 PointsError using lambda
String It = (String one, String two) -> { return "Hello"; };
getting error: target type of lambda conversion must be an interface.
what am i doing wrong??
1 Answer
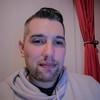
Daniel Hartin
5,311 PointsHi Chanda,
You can't just use Lambda expressions anywhere in your code. It has to be on a method that is defined in an interface with just a single method.
If you have done any Java or Android programming with a GUI you will have come across OnClickListener or something of the sort. OnClickListener isn't a normal class as such even though you instantiate it as one instead an interface is like a rule set for classes that implement the interface meaning that unrelated classes can send messages to one another without the need for inheritance as Java only allows clases to inherit from one parent class and this doesn't work well for object orientated principles all the time.
Lambdas can be used when the rules(interface) say that a class MUST implement a single method this is becuase the compiler doesn't have to guess anything you must be implementing that method and it must have the same signature as defined in the interface so it makes sense to simply leave all this out and this is what Craig explains.
In order for you to use your own you ahve to create an Interface similar to this
public interface RuleSetter{
public void thisMethodMustbeCalled();
}
public class ANormalClass {
RuleSetter ruleSetter;
public setRuleSetter(RuleSetter r){
this.ruleSetter = r;
}
}
public class AnotherClass() implements RuleSetter{
private void init(){
ANormalClass c = new ANormalClass();
c.setRuleSetter(this);
c.setRuleSetter(new RulerSetter() ->{
//I also have to follow the rules but as there's only rule I have to follow.... you can work it out
});
}
public void thisMethodMustBeCalled(){
//Do somethine important!!!
//I have to follow the rules and have a method that is public void thisMethodMustBeCalled
}
}
In both methods where I call setRuleSetter on ANormalClass the RuleSetter object I pass in MUST implement the method thisMethodMustBeCalled() and it causes a compiler error if it doesn't follow the rules laid down by the Interface. Now you can create a method inside an object the normal way as I have and use
public void thisMethodMustBeCalled(){
//Do somethine important!!!
//I have to follow the rules and have a method that is public void thisMethodMustBeCalled
}
This is perfectly valid, but when there is only one method I can also create an annoymous object which also has to implement this method but there's only one so the compiler can save me the job and just make it itself.
It's kind of hard to explain and I'm not the best but I hope this at least give you an insight
Daniel
Chanda Lupambo
Courses Plus Student 27,336 PointsChanda Lupambo
Courses Plus Student 27,336 PointsThanks Daniel, I never used interfaces before, probably why but i started using them and tried using lambdas and it's working. Thanks for the help!