Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial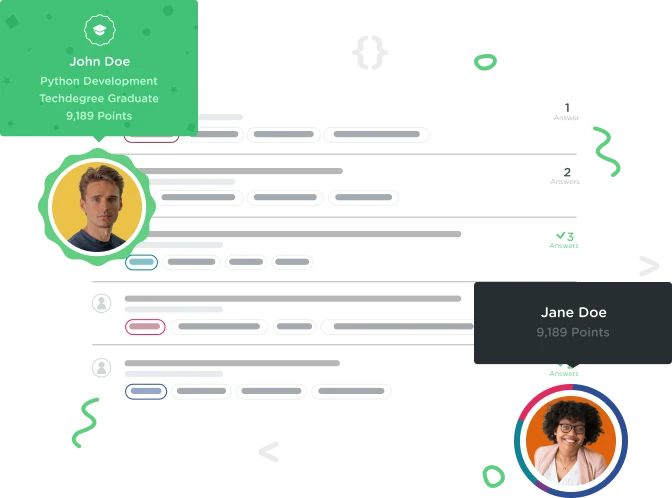

Alemneh Asefa
9,503 PointsError when adding new task:
Uncaught TypeError: Cannot set property 'onchange' of null app.js:111 bindTaskEvents app.js:111 addTask app.js:48
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-task
var completeTasksHolder = document.getElementById("completed-tasks"); //completed-task
//New Task List Item
var createNewTaskElement = function(taskString) {
//Create List Item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input");
//label
var label = document.createElement("label");
//input (text)
var editInput = document.createElement("input");
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//Each element needs modifing
checkBox.type = "input";
label.innerText = taskString;
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
//Each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function() {
console.log("Add task...");
//Create a new list item with the text from #new-task:
var listItem = createNewTaskElement("Some new task");
//Append listItem to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Edit an existing task
var editTask = function() {
console.log("Edit task...");
//When the Edit button is pressed
//if the class of the parent is .editMode
//Switch from .editMode
//label text become the input's value
//else
//Switch to .editMode
//input value becomes the label's text
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
//Remove the parent list item from the ul
ul.removeChild(listItem);
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Task complete...");
//Append the task list to the #completed-tasks
var listItem = this.parentNode;
completeTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
//Append the task list to the #incomplete-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
var bindTaskEvents = function( taskListItem, checkBoxEventHandler) {
console.log("Bind list items");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind the editTask to edit button
editButton.onclick = editTask;
//bind the deleteTask to the delete button
deleteButton.onclick = deleteTask;
//bind the checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Cycle over incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++)
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
//Cycle over completeTasksHolder ul list items
for(var i = 0; i < completeTasksHolder.children.length; i++) {
//bind events to list item's children (taskIncomplete)
bindTaskEvents(completeTasksHolder.children[i], taskIncomplete);
}
1 Answer

John Magee
Courses Plus Student 9,058 PointsCamel Case?
Alemneh Asefa
9,503 PointsAlemneh Asefa
9,503 PointsCan you elaborate on that?
John Magee
Courses Plus Student 9,058 PointsJohn Magee
Courses Plus Student 9,058 PointscamelCase versus camelcase