Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial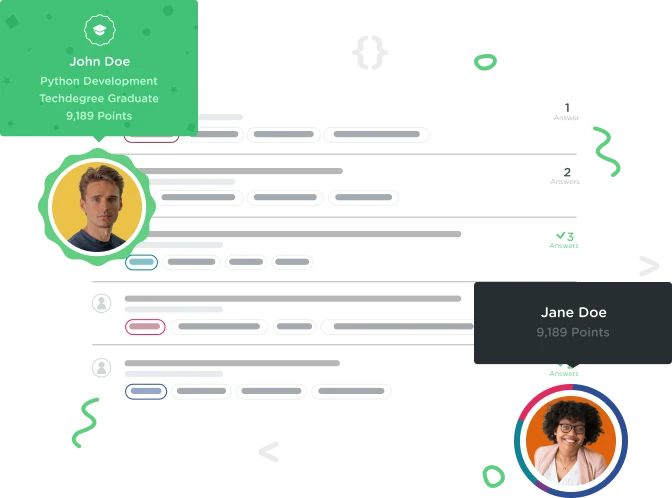
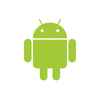
Sebastian Portocarrero Leon
3,871 Pointserror when I tap 7 days...
I have this error in the logCat:
E/AndroidRuntime: FATAL EXCEPTION: main Process: com.sebasportoleon.stormy, PID: 29468 java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.ImageView.setImageResource(int)' on a null object reference at com.sebasportoleon.stormy.addapters.DayAdapter.getView(DayAdapter.java:59) at android.widget.AbsListView.obtainView(AbsListView.java:2344) at android.widget.ListView.makeAndAddView(ListView.java:1864) at android.widget.ListView.fillDown(ListView.java:698) at android.widget.ListView.fillFromTop(ListView.java:759) at android.widget.ListView.layoutChildren(ListView.java:1673) at android.widget.AbsListView.onLayout(AbsListView.java:2148) at android.view.View.layout(View.java:15614) at android.view.ViewGroup.layout(ViewGroup.java:4968) at android.widget.RelativeLayout.onLayout(RelativeLayout.java:1076) at android.view.View.layout(View.java:15614) at android.view.ViewGroup.layout(ViewGroup.java:4968) at android.widget.FrameLayout.layoutChildren(FrameLayout.java:573) at android.widget.FrameLayout.onLayout(FrameLayout.java:508) at android.view.View.layout(View.java:15614) at android.view.ViewGroup.layout(ViewGroup.java:4968) at android.widget.LinearLayout.setChildFrame(LinearLayout.java:1703) at android.widget.LinearLayout.layoutVertical(LinearLayout.java:1557) at android.widget.LinearLayout.onLayout(LinearLayout.java:1466) at android.view.View.layout(View.java:15614) at android.view.ViewGroup.layout(ViewGroup.java:4968) at android.widget.FrameLayout.layoutChildren(FrameLayout.java:573) at android.widget.FrameLayout.onLayout(FrameLayout.java:508) at android.view.View.layout(View.java:15614) at android.view.ViewGroup.layout(ViewGroup.java:4968) at android.view.ViewRootImpl.performLayout(ViewRootImpl.java:2102) at android.view.ViewRootImpl.performTraversals(ViewRootImpl.java:1859) at android.view.ViewRootImpl.doTraversal(ViewRootImpl.java:1077) at android.view.ViewRootImpl$TraversalRunnable.run(ViewRootImpl.java:5884) at android.view.Choreographer$CallbackRecord.run(Choreographer.java:767) at android.view.Choreographer.doCallbacks(Choreographer.java:580) at android.view.Choreographer.doFrame(Choreographer.java:550) at android.view.Choreographer$FrameDisplayEventReceiver.run(Choreographer.java:753) at android.os.Handler.handleCallback(Handler.java:739) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:135) at android.app.ActivityThread.main(ActivityThread.java:5312) at java.lang.reflect.Method.invoke(Native Method) at java.lang.reflect.Method.invoke(Method.java:372) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:901) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:696)
I dont know how to fix it Day adapter code:
package com.sebasportoleon.stormy.addapters;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.sebasportoleon.stormy.R;
import com.sebasportoleon.stormy.weather.Day;
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0; // we aren't going to use this. Tag items for easy reference
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
// brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
if (position == 0) {
holder.dayLabel.setText("Today");
}
else {
holder.dayLabel.setText(day.getDayOfTheWeek());
}
return convertView;
}
private static class ViewHolder {
ImageView iconImageView; // public by default
TextView temperatureLabel;
TextView dayLabel;
}
}
3 Answers

Gavin Ralston
28,770 PointsSooo...
java.lang.NullPointerException:
Attempt to invoke virtual method
'void android.widget.ImageView.setImageResource(int)'
on a null object reference
at com.sebasportoleon.stormy.addapters.DayAdapter.getView(DayAdapter.java:59)
So reading the stack trace, I'm seeing that somewhere inside the DayAdapter class is the getView() method which got called, and inside that method the setImageResource() method is being called from an ImageView you're working with, except that it's expecting an int but it's getting null instead.
This is probably line 59 (or thereabouts) in your DayAdapter class.
holder.iconImageView.setImageResource(day.getIconId());
Looks like day.getIconId() isn't returning a value that you can use right now.
Remember how earlier on in this course there was a refactoring of the Day class getIconId() method when the getIconID() method was added as a static method on the Forecast class?
Make sure you updated it to include the following:
public int getIconId() {
return Forecast.getIconId(mIcon);
}
Rather than it just returning null or something. If that looks okay as well, be sure your Forecast.getIconId() method is doing what you want it to, which should be returning an int, and not like return null or some other auto-generated code when you first created the method with an alt-enter quick fix or shortcut or something,.
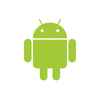
Sebastian Portocarrero Leon
3,871 PointsI already done that but the error persist

Gavin Ralston
28,770 PointsSorry I couldn't be more help.
If the error is still the same, then without a doubt the setImageResource() method is being called on a null value instead of an integer for the android resource ID like you want it to.
You'll want to keep examining whatever you're doing to pass that value in and you'll find your issue.
Feel free to post any and all code related to the project. Specifically the Day class and the Forecast class.
Otherwise, that's the best I can do just guessing. :)

Abhinav Kanoria
7,730 PointsDoes the problem still persist? Maybe you should put the images in the drawable folder in the res directory instead of the mipmap folder (not sure if it makes a difference). And if the error had been sorted out, could you tell me what had gone wrong and how you fixed it?
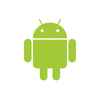
Sebastian Portocarrero Leon
3,871 PointsThanks for the help here is the code
Day, Forecast and MainActivity
public class Day implements Parcelable {
private long mTime;
private String mSummary;
private double mTemperatureMax;
private String mIcon;
private String mTimezone;
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public int getTemperatureMax() {
return (int)Math.round(mTemperatureMax);
}
public void setTemperatureMax(double temperatureMax) {
mTemperatureMax = temperatureMax;
}
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public int getIconId() {
return Forecast.getIconId(mIcon);
}
public String getDayOfTheWeek() {
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(mTimezone));
Date dateTime = new Date(mTime * 1000);
return formatter.format(dateTime);
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(mTime);
dest.writeString(mSummary);
dest.writeDouble(mTemperatureMax);
dest.writeString(mIcon);
dest.writeString(mTimezone);
}
private Day(Parcel in) {
mTime = in.readLong();
mSummary = in.readString();
mTemperatureMax = in.readDouble();
mIcon = in.readString();
mTimezone = in.readString();
}
public Day() { }
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel source) {
return new Day(source);
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
}
public class Forecast {
private Current mCurrent;
private Hour[] mHourlyForecast;
private Day[] mDailyForecast;
public Current getCurrent() {
return mCurrent;
}
public void setCurrent(Current current) {
mCurrent = current;
}
public Hour[] getHourlyForecast() {
return mHourlyForecast;
}
public void setHourlyForecast(Hour[] hourlyForecast) {
mHourlyForecast = hourlyForecast;
}
public Day[] getDailyForecast() {
return mDailyForecast;
}
public void setDailyForecast(Day[] dailyForecast) {
mDailyForecast = dailyForecast;
}
public static int getIconId(String iconString){
//clear-day, clear-night, rain, snow, sleet, wind, fog, cloudy, partly-cloudy-day, or partly-cloudy-night
int iconId = R.mipmap.clear_day;
if (iconString.equals("clear-day")) {
iconId = R.mipmap.clear_day;
}
else if (iconString.equals("clear-night")) {
iconId = R.mipmap.clear_night;
}
else if (iconString.equals("rain")) {
iconId = R.mipmap.rain;
}
else if (iconString.equals("snow")) {
iconId = R.mipmap.snow;
}
else if (iconString.equals("sleet")) {
iconId = R.mipmap.sleet;
}
else if (iconString.equals("wind")) {
iconId = R.mipmap.wind;
}
else if (iconString.equals("fog")) {
iconId = R.mipmap.fog;
}
else if (iconString.equals("cloudy")) {
iconId = R.mipmap.cloudy;
}
else if (iconString.equals("partly-cloudy-day")) {
iconId = R.mipmap.partly_cloudy;
}
else if (iconString.equals("partly-cloudy-night")) {
iconId = R.mipmap.cloudy_night;
}
return iconId;
}
}
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
public static final String DAILY_FORECAST = "DAILY_FORECAST";
public static final String HOURLY_FORECAST = "HOURLY_FORECAST";
private Forecast mForecast;
@Bind(R.id.timeLabel) TextView mTimeLabel;
@Bind(R.id.temperatureLabel) TextView mTemperatureLabel;
@Bind(R.id.humidityValue) TextView mHumidityValue;
@Bind(R.id.precipValue) TextView mPrecipValue;
@Bind(R.id.summaryLabel) TextView mSummaryLabel;
@Bind(R.id.iconImageView) ImageView mIconImageView;
@Bind(R.id.refreshImageView) ImageView mRefreshImageView;
@Bind(R.id.progressBar) ProgressBar mProgressBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
mProgressBar.setVisibility(View.INVISIBLE);
final double latitude = 37.8267;
final double longitude = -122.423;
mRefreshImageView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
getForecast(latitude, longitude);
}
});
getForecast(latitude, longitude);
Log.d(TAG, "Main UI code is running!");
}
private void getForecast(double latitude, double longitude) {
String apiKey = "27974c4bc33201748eaf542a6769c3b7";
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey +
"/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
toggleRefresh();
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
alertUserAboutError();
}
@Override
public void onResponse(Response response) throws IOException {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mForecast = parseForecastDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else {
Toast.makeText(this, getString(R.string.network_unaviable_message),
Toast.LENGTH_LONG).show();
}
}
private void toggleRefresh() {
if (mProgressBar.getVisibility() == View.INVISIBLE) {
mProgressBar.setVisibility(View.VISIBLE);
mRefreshImageView.setVisibility(View.INVISIBLE);
}
else {
mProgressBar.setVisibility(View.INVISIBLE);
mRefreshImageView.setVisibility(View.VISIBLE);
}
}
private void updateDisplay() {
Current current = mForecast.getCurrent();
mTemperatureLabel.setText(current.getTemperature() + "");
mTimeLabel.setText("At " + current.getFormattedTime() + " it will be");
mHumidityValue.setText(current.getHumidity() + "");
mPrecipValue.setText(current.getPrecipChance() + "%");
mSummaryLabel.setText(current.getSummary());
Drawable drawable = getResources().getDrawable(current.getIconId());
mIconImageView.setImageDrawable(drawable);
}
private Forecast parseForecastDetails(String jsonData) throws JSONException {
Forecast forecast = new Forecast();
forecast.setCurrent(getCurrentDetails(jsonData));
forecast.setHourlyForecast(getHourlyForecast(jsonData));
forecast.setDailyForecast(getDailyForecast(jsonData));
return forecast;
}
private Day[] getDailyForecast(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject daily = forecast.getJSONObject("daily");
JSONArray data = daily.getJSONArray("data");
Day[] days = new Day[data.length()];
for (int i = 0; i < data.length(); i++) {
JSONObject jsonDay = data.getJSONObject(i);
Day day = new Day();
day.setSummary(jsonDay.getString("summary"));
day.setIcon(jsonDay.getString("icon"));
day.setTemperatureMax(jsonDay.getDouble("temperatureMax"));
day.setTime(jsonDay.getLong("time"));
day.setTimezone(timezone);
days[i] = day;
}
return days;
}
private Hour[] getHourlyForecast(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject hourly = forecast.getJSONObject("hourly");
JSONArray data = hourly.getJSONArray("data");
Hour[] hours = new Hour[data.length()];
for (int i = 0; i < data.length(); i++) {
JSONObject jsonHour = data.getJSONObject(i);
Hour hour = new Hour();
hour.setSummary(jsonHour.getString("summary"));
hour.setIcon(jsonHour.getString("icon"));
hour.setTemperature(jsonHour.getDouble("temperature"));
hour.setTime(jsonHour.getLong("time"));
hour.setTimezone(timezone);
hours[i] = hour;
}
return hours;
}
private Current getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currently");
Current current = new Current();
current.setHumidity(currently.getDouble("humidity"));
current.setTime(currently.getLong("time"));
current.setIcon(currently.getString("icon"));
current.setPrecipChance(currently.getDouble("precipProbability"));
current.setSummary(currently.getString("summary"));
current.setTemperature(currently.getDouble("temperature"));
current.setTimeZone(timezone);
Log.d(TAG, current.getFormattedTime());
return current;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
@OnClick (R.id.dailyButton)
public void startDailyActivity(View view) {
Intent intent = new Intent(this, DailyForecastActivity.class);
intent.putExtra(DAILY_FORECAST, mForecast.getDailyForecast());
startActivity(intent);
}
@OnClick (R.id.hourlyButton)
public void startHourlyActivity(View view){
Intent intent = new Intent(this, DailyForecastActivity.class);
intent.putExtra(HOURLY_FORECAST,mForecast.getHourlyForecast());
startActivity(intent);
}
}