Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial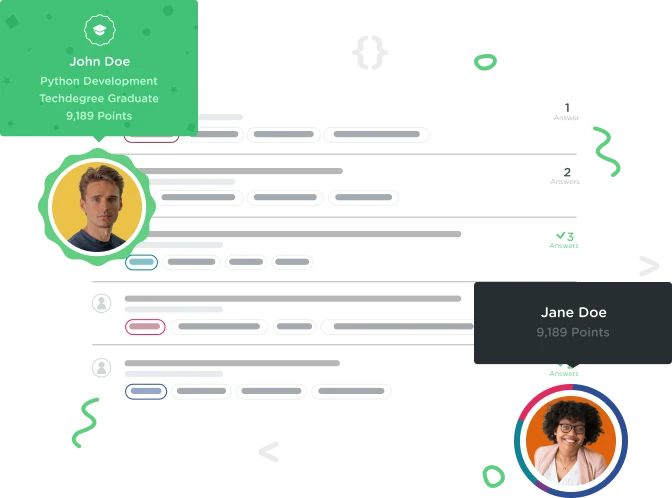

Jason Herscovici
8,683 PointsError when loading into browser
const players = [
{
name: "Guil",
score: 50
},
{
name: "Treasure",
score: 85
},
{
name: "Ashley",
score: 95
},
{
name: "James",
score: 80
}
];
const Header = (props) => {
console.log(props)
return (
<header>
<h1>{ props.title }</h1>
<span className="stats">Players: { props.totalPlayers }</span>
</header>
);
}
const Player = (props) => {
return (
<div className="player">
<span className="player-name">
{ props.name }
</span>
<Counter score={props.score} />
</div>
);
}
const Counter = (props) => {
return (
<div className="counter">
<button className="counter-action decrement"> - </button>
<span className="counter-score"> {props.score} </span>
<button className="counter-action increment"> + </button>
</div>
);
}
const App = (props) => {
return (
<div className="Scoreboard">
<Header title="Scoreboard" totalPlayers={1}/>
{props.initialPlayers.map( player =>
<Player
name={player.name}
score={player.score}
/>
)}
</div>
);
}
ReactDOM.render(
<App intialPlayers={players} />,
document.getElementById('root')
);
Not sure what's wrong here? This isn't loading and I'm getting the following error:
react.development.js:2337 [Deprecation] SharedArrayBuffer will require cross-origin isolation as of M91, around May 2021. See https://developer.chrome.com/blog/enabling-shared-array-buffer/ for more details. (anonymous) @ react.development.js:2337 (anonymous) @ react.development.js:15 (anonymous) @ react.development.js:16 babel.min.js:24 You are using the in-browser Babel transformer. Be sure to precompile your scripts for production - https://babeljs.io/docs/setup/ u @ babel.min.js:24 f @ babel.min.js:1 (anonymous) @ babel.min.js:1 app.js:56 Uncaught TypeError: Cannot read property 'map' of undefined at App (<anonymous>:78:30) at renderWithHooks (react-dom.development.js:14938) at mountIndeterminateComponent (react-dom.development.js:17617) at beginWork (react-dom.development.js:18731) at HTMLUnknownElement.callCallback (react-dom.development.js:182) at Object.invokeGuardedCallbackDev (react-dom.development.js:231) at invokeGuardedCallback (react-dom.development.js:286) at beginWork$1 (react-dom.development.js:23338) at performUnitOfWork (react-dom.development.js:22289) at workLoopSync (react-dom.development.js:22265) App @ app.js:56 renderWithHooks @ react-dom.development.js:14938 mountIndeterminateComponent @ react-dom.development.js:17617 beginWork @ react-dom.development.js:18731 callCallback @ react-dom.development.js:182 invokeGuardedCallbackDev @ react-dom.development.js:231 invokeGuardedCallback @ react-dom.development.js:286 beginWork$1 @ react-dom.development.js:23338 performUnitOfWork @ react-dom.development.js:22289 workLoopSync @ react-dom.development.js:22265 performSyncWorkOnRoot @ react-dom.development.js:21891 scheduleUpdateOnFiber @ react-dom.development.js:21323 updateContainer @ react-dom.development.js:24508 (anonymous) @ react-dom.development.js:24893 unbatchedUpdates @ react-dom.development.js:22038 legacyRenderSubtreeIntoContainer @ react-dom.development.js:24892 render @ react-dom.development.js:24975 (anonymous) @ app.js:69 i @ babel.min.js:24 r @ babel.min.js:24 e.src.n.<computed>.l.content @ babel.min.js:24 n.onreadystatechange @ babel.min.js:24 XMLHttpRequest.send (async) s @ babel.min.js:24 (anonymous) @ babel.min.js:24 o @ babel.min.js:24 u @ babel.min.js:24 f @ babel.min.js:1 (anonymous) @ babel.min.js:1 react_devtools_backend.js:2556 The above error occurred in the <App> component: in App
Consider adding an error boundary to your tree to customize error handling behavior. Visit https://fb.me/react-error-boundaries to learn more about error boundaries. overrideMethod @ react_devtools_backend.js:2556 logCapturedError @ react-dom.development.js:19662 logError @ react-dom.development.js:19699 update.callback @ react-dom.development.js:20843 callCallback @ react-dom.development.js:12625 commitUpdateQueue @ react-dom.development.js:12646 commitLifeCycles @ react-dom.development.js:20018 commitLayoutEffects @ react-dom.development.js:22938 callCallback @ react-dom.development.js:182 invokeGuardedCallbackDev @ react-dom.development.js:231 invokeGuardedCallback @ react-dom.development.js:286 commitRootImpl @ react-dom.development.js:22676 unstable_runWithPriority @ react.development.js:2685 runWithPriority$1 @ react-dom.development.js:11174 commitRoot @ react-dom.development.js:22516 finishSyncRender @ react-dom.development.js:21942 performSyncWorkOnRoot @ react-dom.development.js:21928 scheduleUpdateOnFiber @ react-dom.development.js:21323 updateContainer @ react-dom.development.js:24508 (anonymous) @ react-dom.development.js:24893 unbatchedUpdates @ react-dom.development.js:22038 legacyRenderSubtreeIntoContainer @ react-dom.development.js:24892 render @ react-dom.development.js:24975 (anonymous) @ app.js:69 i @ babel.min.js:24 r @ babel.min.js:24 e.src.n.<computed>.l.content @ babel.min.js:24 n.onreadystatechange @ babel.min.js:24 XMLHttpRequest.send (async) s @ babel.min.js:24 (anonymous) @ babel.min.js:24 o @ babel.min.js:24 u @ babel.min.js:24 f @ babel.min.js:1 (anonymous) @ babel.min.js:1 react-dom.development.js:22800 Uncaught TypeError: Cannot read property 'map' of undefined at App (<anonymous>:78:30) at renderWithHooks (react-dom.development.js:14938) at mountIndeterminateComponent (react-dom.development.js:17617) at beginWork (react-dom.development.js:18731) at HTMLUnknownElement.callCallback (react-dom.development.js:182) at Object.invokeGuardedCallbackDev (react-dom.development.js:231) at invokeGuardedCallback (react-dom.development.js:286) at beginWork$1 (react-dom.development.js:23338) at performUnitOfWork (react-dom.development.js:22289) at workLoopSync (react-dom.development.js:22265)
2 Answers

Blake Larson
13,014 PointsThis works for me in the browser if I make a react project, but can act different in the workspaces. If you can't make it work just use the players
array variable right in the App
component and don't pass any props to that component. Like this..
const players = [
{
name: "Guil",
score: 50
},
{
name: "Treasure",
score: 85
},
{
name: "Ashley",
score: 95
},
{
name: "James",
score: 80
}
];
const Header = (props) => {
console.log(props)
return (
<header>
<h1>{ props.title }</h1>
<span className="stats">Players: { props.totalPlayers }</span>
</header>
);
}
const Player = (props) => {
return (
<div className="player">
<span className="player-name">
{ props.name }
</span>
<Counter score={props.score} />
</div>
);
}
const Counter = (props) => {
return (
<div className="counter">
<button className="counter-action decrement"> - </button>
<span className="counter-score"> {props.score} </span>
<button className="counter-action increment"> + </button>
</div>
);
}
const App = (props) => {
return (
<div className="Scoreboard">
<Header title="Scoreboard" totalPlayers={players.length}/>
{players.map( player =>
<Player
name={player.name}
score={player.score}
/>
)}
</div>
);
}
ReactDOM.render(
<App />,
document.getElementById('root')
);
As long as the players array is in the same file as the App component this way works.
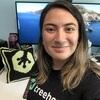
Laura Coronel
Treehouse TeacherAwesome work so far Jason Herscovici! While taking a look at your code it looks like at line 70 you have a small typo. You are missing the second "i" in initialPlayers
.
<App intialPlayers={players} />,
should be
<App initialPlayers={players} />,
Typos are very common mistakes that a lot of developers make.
I also do want to point out in line 53 you have the class name "Scoreboard" capitalized when it should be "scoreboard" so that it matches the css classname.
Keep up the hard work!