Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial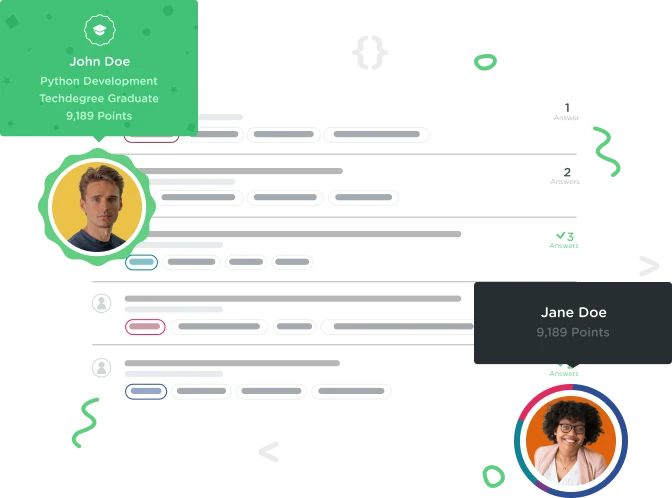

sairam poruri
Python Web Development Techdegree Student 3,213 PointsError while creating dice class
Hi,
I get an error while writing the code for the given problem. It says that it can't get the length of the hand but I am inheriting a list which should have inherently have the method present. I have tried creating the len method explicitly too but it did not work.
Please help.
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(20)
import dice
class Hand(list):
def __init__(self):
super().__init__()
@property
def total(self):
return sum(self)
def roll(self,cont):
for _ in range(cont):
self.append(dice.D20())
return self
1 Answer
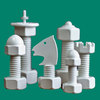
Steven Parker
229,732 PointsThe instructions say, "I'm going to use code similar to Hand.roll(2)
...". Notice that the method is being called on the class name (Hand
) instead of an instance, so this is a clue that "roll" should probably be a class method. And it also said, "I want to get back an instance of Hand..." which is a clue that a new instance should be created by the method and passed back.
sairam poruri
Python Web Development Techdegree Student 3,213 Pointssairam poruri
Python Web Development Techdegree Student 3,213 PointsHi Steven,
Thank you so much for your help. But I am not able to implement what you said!! Can you point out the mistake that I am making here in this code......
Steven Parker
229,732 PointsSteven Parker
229,732 PointsThere's no "self" in a class method, and you still need to create a new instance, append to it, and return it:
Also, you don't need to override "
__init__
".