Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial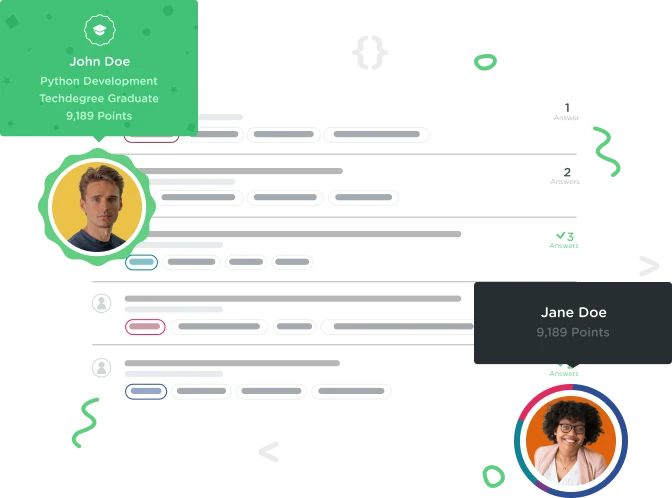

Tojo Alex
Courses Plus Student 13,331 PointsError with code challenge
I keep on getting these error's so I don't know what i'm doing:
StudentsCode.cs(12,25): error CS1525: Unexpected symbol 22'
StudentsCode.cs(12,27): warning CS0642: Possible mistaken empty statement
StudentsCode.cs(12,27): error CS1525: Unexpected symbol
)'
StudentsCode.cs(15,0): error CS1525: Unexpected symbol else'
StudentsCode.cs(15,23): error CS1525: Unexpected symbol
{'
Compilation failed: 4 error(s), 1 warnings
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature <= 21){
Console.WriteLine("Too cold!");
}
else if(temperature = 21 22){
Console.WriteLine("Just right.");
}
else(temperature >= 22){
Console.WriteLine("Too hot!");
}
1 Answer

andren
28,558 PointsThere are a couple of issues with your code.
"Too cold" should only be printed when temperature
is less than 21, you print it if temperature
is less than or equal to 21.
In this line of code:
else if(temperature = 21 22){
There are two issues. The first is that you have used the assigned operator (=
) rather than the equality comparison operator (==
) when checking the number. The second issue is that you can't compare multiple numbers just by typing them in a row like you have done. If you have multiple numbers you need to compare then each number will need it's own condition. In this case you could have two conditions combined with the OR operator (||) like this:
else if(temperature == 21 OR temperature == 22){
But if would be more efficient and clean to simply use a less than or equal to comparison like this:
else if(temperature <= 22){
Since that would achieve the same effect.
The final issue is that else
is a statement that automatically runs if the if/else if
condition it is connected to did not run. Passing it conditions within parenthesis is actually invalid, which is why your code does not compile. You can solve that issue but simply removing the conditions, the else
statement will work fine without it since the other temperature ranges are already being covered by the if/else if
above it.
So if you solve all of those issues like this:
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature < 21){
Console.WriteLine("Too cold!");
}
else if(temperature <= 22){
Console.WriteLine("Just right.");
}
else {
Console.WriteLine("Too hot!");
}
Then your code will pass.
Tojo Alex
Courses Plus Student 13,331 PointsTojo Alex
Courses Plus Student 13,331 Pointsthank you i realize what i did wrong