Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial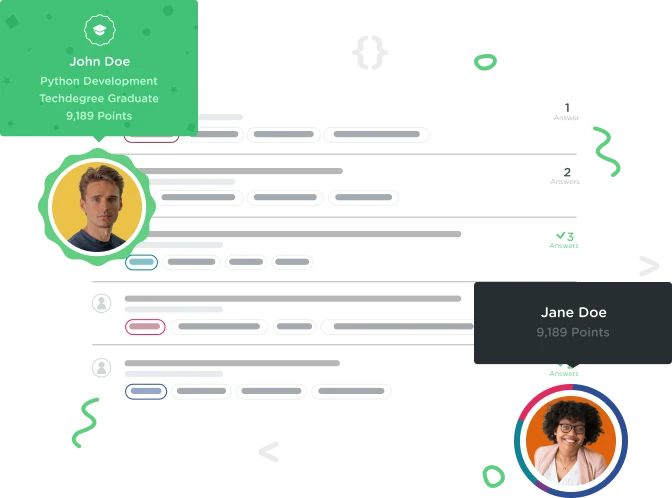

Destiny Robbins
549 PointsError with summing elements in ArrayList.
Here is my code:
import java.io.*;
import java.math.*;
import java.text.*;
import java.util.*;
import java.util.regex.*;
class Fibonacci
{
long f1=0;
long f2=1;
long f3=0;
int start, end;
static ArrayList<Integer> evenFibArrList = new ArrayList<Integer>();
static int evenFibSum = 0;
void get() throws IOException
{
BufferedReader Br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("\nEnter the start limit: ");
start = Integer.parseInt(Br.readLine());
System.out.print("\nEnter the end limit: ");
end = Integer.parseInt(Br.readLine());
System.out.println("\nFibonacci numbers between " + start + " and " + end + " are:" );
}
void display()
{
for (int i = start; i < end; i++)
{
if (f3 <= end)
{
if (f3 >=start)
{
System.out.println(f3);
if (f3 % 2 == 0)
{
evenFibArrList.add(new Integer(f3));
for(int s = 0; s < evenFibArrList.size(); s++)
{
//evenFibSum += evenFibArrList.get(s);
}
}
}
f1 = f2;
f2 = f3;
f3 = f1 + f2;
}
}
}
public static void main(String args[]) throws IOException
{
Fibonacci fib = new Fibonacci();
fib.get();
fib.display();
System.out.println(evenFibArrList);
System.out.println(evenFibSum);
}
}
I am trying to add the elements of an array list together and print the sum. Here is the error:
Fibonacci.java:42: error: no suitable constructor found for Integer(long)
evenFibArrList.add(new Integer(f3));
^
constructor Integer.Integer(int) is not applicable
(argument mismatch; possible lossy conversion from long to int)
constructor Integer.Integer(String) is not applicable
(argument mismatch; long cannot be converted to String)
1 error
1 Answer

Lukas Dahlberg
53,736 PointsYou've declared f1, f2, and f3 as longs. A long is a 64 bit signed integer (in other words, it can have a +/-) whereas an integer is only 32 bits. So a long will not fit inside an integer while an integer will fit inside a long.
So, when you try to add long to Integer (using autoboxing, incidentally), the compiler throws a fit because you didn't cast long to int, explicitly telling the compiler to do what it can to get an long inside an int, even if the program has to throw away extra bits which, as you might tell, is a bad idea. Instead, you should use List<Long> if you want to keep f1, f2, f3 as long or you should change f1, f2, f3 to int.