Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial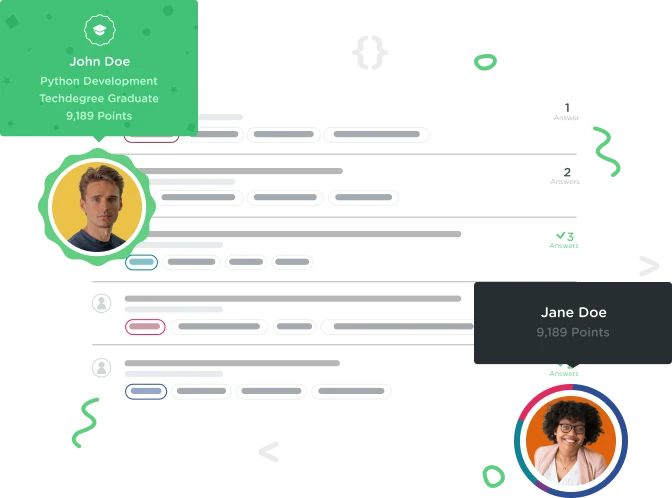
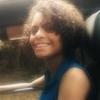
Amelia Groen
6,144 PointsError: write after end - ONLY when using 'Content-Type': 'text/html'
If I go to a username page my server stops running and I get an Error: write after end, but only when I change the content type to text/html, it works fine when I'm using text/plain.
My code - router:
const Profile = require("./profile.js");
const renderer = require('./renderer.js');
const commonHeaders = {'Content-Type': 'text/html'};
// Handle HTTP route GET / and POST / i.e. Home
function home(request, response) {
// if url === "/" && GET
if (request.url === '/') {
//show search field
response.writeHead(200, commonHeaders);
renderer.view('header', {}, response);
renderer.view('search', {}, response);
renderer.view('footer', {}, response);
response.end();
}
// if url === "/" && POST
// redirect to /:username
}
// Handle HTTP route GET / :username i.e. /ameliagroen
function user(request, response) {
// if url === "/..."
const username = request.url.replace('/', '');
if (username.length > 0) {
response.writeHead(200, commonHeaders);
renderer.view('header', {}, response);
// get json from Treehouse
const studentProfile = new Profile(username);
// on 'end'
studentProfile.on("end", profileJSON => {
// show profile
// store values which we need
const values = {
avatarURL: profileJSON.gravatar_url,
username: profileJSON.name,
badges: profileJSON.badges.length,
javascriptPoints: profileJSON.points.JavaScript
}
// Smple response
renderer.view('profile', values, response);
renderer.view('footer', {}, response);
response.end();
});
// on 'error'
studentProfile.on("error", error => {
// show error
renderer.view('error', {errorMessage: error.message}, response);
renderer.view('search', {}, response);
renderer.view('footer', {}, response);
response.end();
});
}
}
module.exports.home = home;
module.exports.user = user;
renderer:
// Function that handles the reading of files and merge in value
const fs = require('fs');
function mergeValues(values, content) {
// cycle over the keys
for(let key in values) {
// replace all {{key}} with the value from the values object
content = content.replace(`{{${key}}}`, values[key]);
}
// return merged content
return content;
}
function view(templateName, values, response) {
// read from the template file
let fileContents = fs.readFileSync(`./views/${templateName}.html`, {encoding: 'utf8'});
// insert values into the content
fileContents = mergeValues(values, fileContents);
// write out the contents to the response
response.write(fileContents);
}
module.exports.view = view;
app:
const router = require('./router.js');
// Problem: We need a simple way to look at a user's badge count and JavaScript points from a web browser
// Solution: Use node.js to perform the profile look ups and serve our template via HTTP
// Create a web server
const http = require('http');
const hostname = '127.0.0.1';
const port = 3001;
const server = http.createServer((request, response) => {
router.home(request, response);
router.user(request, response);
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Amelia Groen
6,144 PointsAmelia Groen
6,144 PointsMy error: