Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial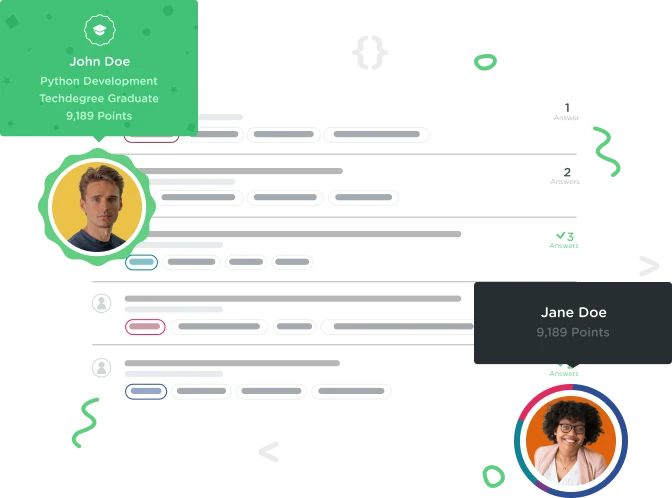
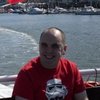
Sean Flanagan
33,235 PointsErrors in code
Hi. I'm not sure what or where the errors are.
package com.teamtreehouse;
import java.io.*;
public class Treets {
public static void save(Treet[] treets) {
try (
FileOutputStream fos = new FileOutputStream("treets.ser");
ObjectOutput Stream oos = new ObjectOutputStream(fos);
) {
oos.writeObject(treets);
} catch(IOException ioe) {
System.out.println("Problem saving Treets");
ioe.printStackTrace();
}
}
}
All the errors are said to be in Treets.java. Here they are:
./com/teamtreehouse/Treets.java:9: error: = expected
ObjectOutput Stream oos = new ObjectOutputStream(fos);
^
./com/teamtreehouse/Treets.java:9: error: '{' expected
ObjectOutput Stream oos = new ObjectOutputStream(fos);
^
./com/teamtreehouse/Treets.java:10: error: illegal start of expression
) {
^
./com/teamtreehouse/Treets.java:12: error: 'catch' without 'try'
} catch(IOException ioe) {
^
./com/teamtreehouse/Treets.java:17: error: reached end of file while parsing
}
^
./com/teamtreehouse/Treets.java:11: error: cannot find symbol
oos.writeObject(treets);
^
symbol: variable oos
location: class Treets
As ever, thanks in advance for any help. :-)
1 Answer

Geoff Parsons
11,679 PointsYou have an extra space on that line so it think you're looking to create an ObjectOutput
variable named Stream
. You need ObjectOutputStream oos
instead:
ObjectOutputStream oos = new ObjectOutputStream(fos);
EDIT: Also looks like you have some extra parenthesis in there around your try / catch.
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi Geoff. Thanks for your reply. I hadn't noticed that space on Line 9 that you mentioned. My program works now. Thank you. :-)
Geoff Parsons
11,679 PointsGeoff Parsons
11,679 PointsSorry for the late reply. Couple more concerns with your code:
As mentioned above the try / catch should be only using curly braces "{ }" like so:
Also I'm assuming your class should be
Treet
notTreets
since you're accepting aTreet
array in the save method. If that's the case the file should also be called Treet.java instead of Treets.java (always name the file after the main public class).Think that should be it to get you compiling. Let me know if you run into any other issues.