Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial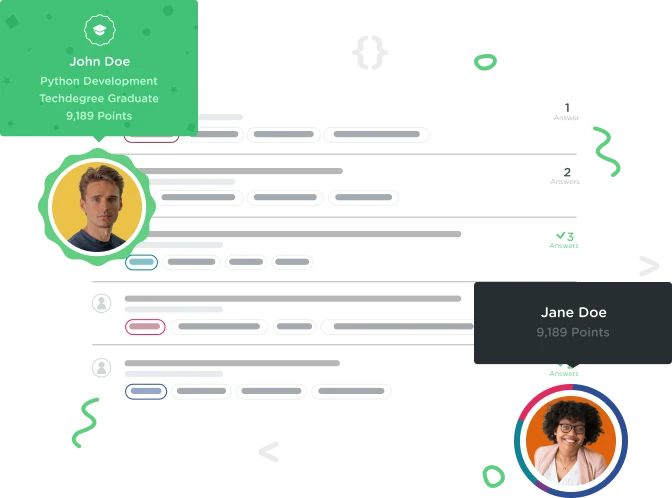

prashant kurup
291 PointsErrors in logcat
02-03 12:22:06.147 29020-29020/teamtreehouse.com.stormy E/AndroidRuntimeοΉ FATAL EXCEPTION: main
Process: teamtreehouse.com.stormy, PID: 29020
java.lang.RuntimeException: Unable to instantiate application android.app.Application: java.lang.IllegalStateException: Unable to get package info for teamtreehouse.com.stormy; is package not installed?
I get this error in the logcat at this stage. Here is my code for the class. I had to change the code in the catch block to catch(Exception e) instead of catch (IOException e) because it kept throwing an error saying that the IOException is never thrown. At this point the app seems to load up on the screen but im not receiving any data. What am i doing wrong?
public class MainActivity extends ActionBarActivity {
public static final String TAG = MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "f7702b51a41e2ed4971569213333ded4";
double latitude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/"+apiKey+"/"+latitude+"," +longitude;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
if (response.isSuccessful()) {
Log.v(TAG, response.body().toString());
}
}
catch (Exception e){
Log.e(TAG, "Exception Caught: ", e);
}
}
});
}
}

prashant kurup
291 PointsHi David, Thanks for your answer. I've managed to fix it after a lot of trial and error. it was a small syntax error. I'm wondering whether i shoudl leave this post be since it might not be useful to anybody else. Mods what say? should i delete the post for being non constructive?
1 Answer
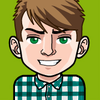
Harry James
14,780 PointsHey Prashant!
This error message is brought up by the Android System when it is unable to close down and reinstall the application (Because you have a previous version of the app already open).
To solve this, all you need to do is close down the app before pressing the "Run" button. If that doesn't fix it, go to Settings and Apps, select the app and press Force Close and try again.
Hope it helps :)
daviddavis3
4,904 Pointsdaviddavis3
4,904 PointsAre all the import statements there - such as the import for ActionBarActivity, and the import of the package at the very top?
Also, check the AndroidManifest.xml to see if the INTERNET permissions are there. I also got errors until I put ACCESS_NETWORK_STATE permission in there.