Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial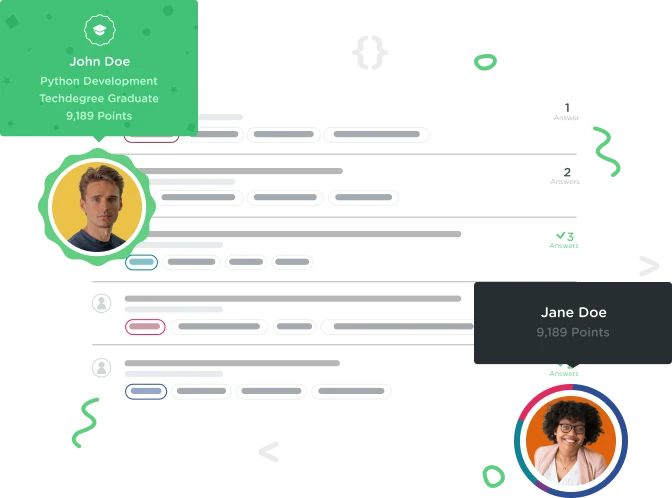

Azzan Al-Rahbi
1,412 Pointserrors java:27,28,29
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String ageAsString = console.readLine("how old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//insert exit code
console.printf("sorry you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Enter a name: ");
String adjective = console.readLine("Enter an adjective: ");
do {
String noun = console.readLine("Enter a noun: ");
if (noun.equalsIgnoreCase("dork") ||
noun.equalsIgnoreCase("jerk"))
console.printf("that language is not allowed. Try again. \n\n");
}
} while(noun.equalsIgnoreCase("dork") ||
noun.equalsIgnoreCase("jerk"));
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb ending with -ing: ");
console.printf("Your TreeStory:\n--------------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
console.printf("They are always %s %s.\n", adverb, verb);
}
}
2 Answers
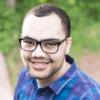
Philip Gales
15,193 PointsAfter formatting your code, I found a few problems.
- Your if statement inside of your do while loop had a closing curly brace with no opening curly brace.
- You declared a variable called "noun" in your do while loop and tried using it in your while statement. Your noun variable is now out of scope when calling it in your while statement giving you an error. To fix this, I declared the noun variable outside of the do while.
Here is your completed code.
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String ageAsString = console.readLine("how old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//insert exit code
console.printf("sorry you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Enter a name: ");
String adjective = console.readLine("Enter an adjective: ");
//declared noun here so we can use it in the while statement.
String noun;
do {
noun = console.readLine("Enter a noun: ");
if (noun.equalsIgnoreCase("dork") || noun.equalsIgnoreCase("jerk")) {
console.printf("that language is not allowed. Try again. \n\n");
}
} while (noun.equalsIgnoreCase("dork") || noun.equalsIgnoreCase("jerk"));
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb ending with -ing: ");
console.printf("Your TreeStory:\n--------------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
console.printf("They are always %s %s.\n", adverb, verb);
} //end of main()

kirankalathil
1,079 PointsCheck if your are missing a brace in your code
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsEDIT: Starting at 2:40 in the video he talks about scope and moves the noun variable as I did.
Azzan Al-Rahbi
1,412 PointsAzzan Al-Rahbi
1,412 Pointsi'am having more errors now
Azzan Al-Rahbi
1,412 PointsAzzan Al-Rahbi
1,412 Pointsi think the problem with workspases i can't save my work
Azzan Al-Rahbi
1,412 PointsAzzan Al-Rahbi
1,412 Pointsthanks man it was a problem with workspases and its done now