Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial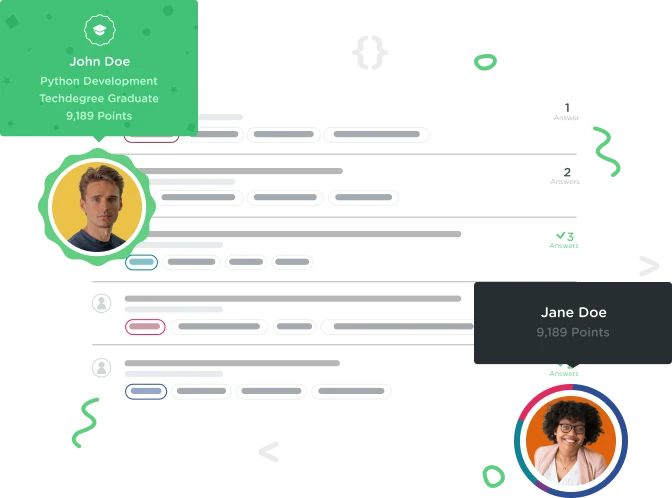
Nick Vitsinsky
7,246 PointsErrors with method on string called contains: 1) boolean cannot be dereferenced; 2) error ';' is expected;
Here are the codes for both examples
boolean isInvalidWord;
do {
noun = console.readLine("Type a noun: ");
isInvalidWord.contains("dork", "jerk");
if (isInvalidWord) {
console.printf("Name Dork is not applicable, you, DORK! Try again. \n\n");
}
} while (isInvalidWord);
it leads me to "boolean cannot be dereferenced"
and
boolean isInvalidWord;
do {
noun = console.readLine("Type a noun: ");
isInvalidWord contains("dork", "jerk");
if (isInvalidWord) {
console.printf("Name Dork is not applicable, you, DORK! Try again. \n\n");
}
} while (isInvalidWord);
leads me to "error: ; 'is' is expected"
What am I doing wrong?
7 Answers
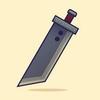
Allan Clark
10,810 PointsJust taking a quick look over your code (haven't run tests on it). isInvalidWord is a boolean not a string, so it does not have the contains method. Likely what you are trying to do is call the contains method on the noun variable and set isInvalidWord to the result. Try this
isInvalidWord = noun.contains("dork", "jerk");

Stephen Emery
14,384 PointsYour method seems to be different than what is taught. Try:
isInvalidWord = (noun.equalsIgnoreCase("dork") || noun.equalsIgnoreCase("jerk"));
Nick Vitsinsky
7,246 PointsSo I understand if variable is boolean I cannot search within it? but if I assign it to another variable (in this case "noun") I can? confused....
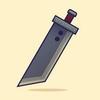
Allan Clark
10,810 PointsA boolean variable can only hold true or false. Since it can only hold these two values, there is no need to search within the variable. 'noun' is a String variable so it gets the .contains method. The contains method returns a boolean value, which is why you will want to check if noun contains dork/jerk and assign what is returned to isInvalidWord.
Nick Vitsinsky
7,246 PointsThanks for your answers
Nick Vitsinsky
7,246 PointsAlright, so I've tried
isInvalidWord = noun.contains("dork", "jerk");
TreeStory.java:26: error: method contains in class String cannot be applied to given types;
isInvalidWord = noun.contains("dork", "jerk");
^
required: CharSequence
found: String,String
reason: actual and formal argument lists differ in length
1 error
I've also tried
isInvalidWord = noun.containsIgnoreCase("dork", "jerk", "dorkjerk", "jerkdork");
as per http://stackoverflow.com/questions/14018478/string-contains-ignore-case and got the following error
TreeStory.java:27: error: cannot find symbol
isInvalidWord = noun.containsIgnoreCase("dork", "jerk", "dorkjerk", "jerkdork");
^
symbol: method containsIgnoreCase(String,String,String,String)
location: variable noun of type String
1 error
here is my full code (I've commented out line 26 isInvalidWord = noun.contains("dork", "jerk"); here)
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//Insert exit code
console.printf("Sorry you must be 13 yo or older to use this app\n");
System.exit(0);
}
String name = console.readLine("What is your name? ");
String adjective = console.readLine("Provide an adjective: ");
String noun;
boolean isInvalidWord;
do {
noun = console.readLine("Enter a noun: ");
//isInvalidWord = noun.contains("dork", "jerk");
isInvalidWord = noun.containsIgnoreCase("dork", "jerk", "dorkjerk", "jerkdork");
if (isInvalidWord) {
console.printf("You cannot use that word. Try again \n\n");
}
} while (isInvalidWord);
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb ending with -ing: ");
console.printf("My TreeStory\n-----------------------\n");
console.printf("%s is very %s and the %s\n", name, adjective, noun);
console.printf("while %s is, finaly verb is %s\n", adverb, verb);
}
}
I can go further in my learning, but I really want to try these Extra credits from the lesson
- There is a method on string called contains. It checks to see if one string is contained within the other. This seems like a good way to build a master list of words that are not allowed. Why don't see if what they typed is in a long string of bad words.
- After you get that working, attempt to take case into account. If your list of bad words that you maintain is all lower cased, you can check the lower case version of the input in the bad string. How do you make a string lowercase?

Rian Cervantes
616 PointsSame problem here. Not sure exactly what to do...

Janis Sauss
4,672 PointsI have the same issue. Can anyone explain? Thank you.

Janis Sauss
4,672 PointsI was solve the problem with one bad word. Still trying to figure out with multiple. Is this code ok?
String noun;
boolean isInvalidWord;
do{
noun = console.readLine("Enter a noun: ");
CharSequence ch;
ch=("dork");
isInvalidWord=noun.contains(ch);
if (isInvalidWord) {
console.printf("Be polite. Try again.\n\n");
}
} while (isInvalidWord);