Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial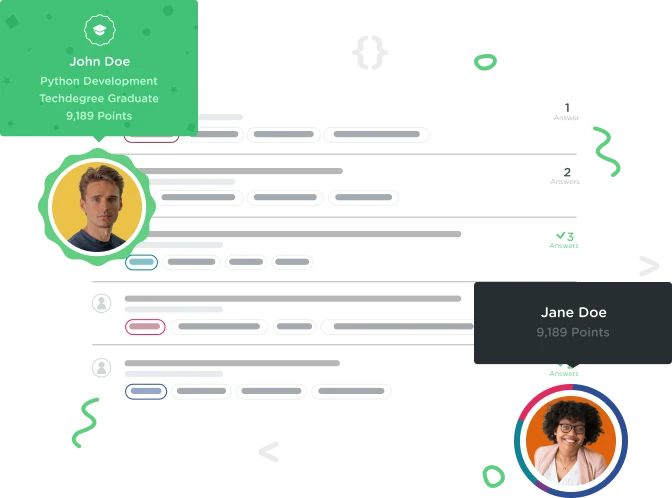
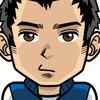
Miguel Barra
6,855 PointsES6 version of profile.js
The code of the video is deprecated, use this version instead. In order to understand EventEmitter, please refers to any video on YouTube explaining EventEmitter in Node.js.
import EventEmitter from 'events';
import { get, statusCode } from 'https';
import { STATUS_CODES } from 'http';
class Profile extends EventEmitter {
constructor(username) {
super();
// need for calling the methods of EventEmitter
let profileInstanceContext = this;
const request = get(`https://teamtreehouse.com/${username}.json`, res => {
let body = '';
if (res.statusCode !== 200) {
request.abort();
profileInstanceContext.emit('error', new Error(`There was an error getting the profile for ${username}. (${STATUS_CODES[statusCode]})`))
}
res.on('data', chunk => {
body += chunk;
profileInstanceContext.emit('data', chunk);
});
res.on('end', () => {
if (res.statusCode === 200) {
try {
// Parse the data
const profile = JSON.parse(body);
profileInstanceContext.emit('end', profile);
} catch (error) {
profileInstanceContext.emit('error', error);
}
}
}).on('error', error => profileInstanceContext.emit('error', error));
});
}
}
export default Profile;
1 Answer
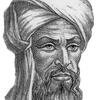
Armend Azemi
27,921 PointsAnother point to notice is that as of 2022, the request URL is wrong, the correct URL to get profiles should now be
let request = https.get(`https://teamtreehouse.com/profiles/${username}.json
Notice that the /profiles/.
Ben McMahan
7,921 PointsBen McMahan
7,921 PointsThis throws an error -