Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial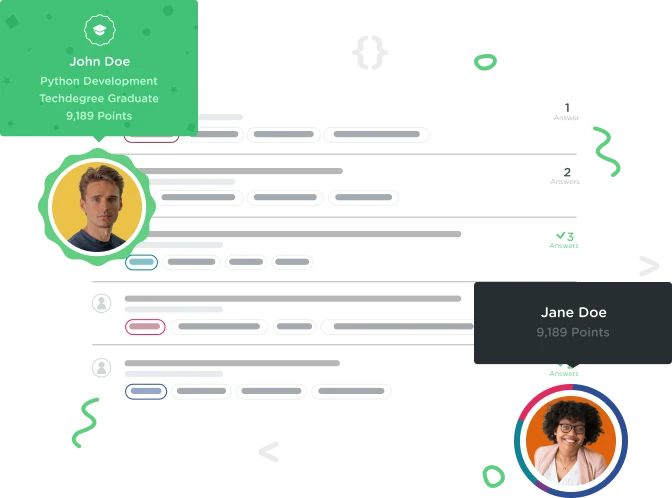

Jaroslaw Imiolek
9,039 PointsEven or odd challenge, step 3 seems to be broken.
Anytime I click Check Work, communication with server breaks.
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while True:
run = random.randint(1, 99)
if run == even_odd:
print('{} is even'.format(run))
else:
print('{} is odd'.format(run))
start -= 1
1 Answer

andren
28,558 PointsThe connection breaks because your code creates an infinite loop. A while loop will run as long as the condition you provide it evaluates to True
, since you are passing it True
directly and have no break
statements in the loop it will loop indefinitively.
The task asks you to make the loop run until start
is falsey. In order to do that you simply need to use the start
variable itself as your looping condition. Also even_odd
is a function, so you need to call it and pass in the randomly generated number, not compare the number and the function directly with an equality comparison.
If you fix those two issues like this:
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start: # Test the "truthiness" of start
run = random.randint(1, 99)
if even_odd(run): # Call even_odd and pass in the random number
print('{} is even'.format(run))
else:
print('{} is odd'.format(run))
start -= 1
Then your code will work.