Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial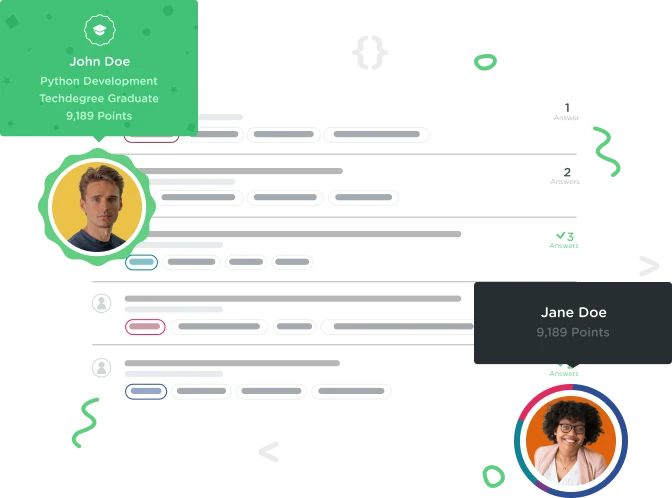

Jason Pallone
895 Pointseven or odd loop challenge help
This is what i got so far, but i cant find where im going wrong?
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start == 5:
num = random.randint(1, 99)
if even_odd(num) % 2 == 0:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
1 Answer
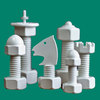
Steven Parker
241,809 PointsYou should not make any changes to the provided "even_odd" function. All your code should be added after (and outside of) the function.
But you can use the function to test your random numbers.
Jason Pallone
895 PointsJason Pallone
895 PointsThanks for the reply, what do you mean by not make any changes to even_odd and having code be outside?
Steven Parker
241,809 PointsSteven Parker
241,809 PointsBecause it is indented, the code you added is all considered to be part of the function. Though it will never be executed because it follows a "return" statement.
To make your code independent from the function, the "while" should begin at the first column.
Also, for testing the number you only need to call the function, you won't need to perform any math with it:
if even_odd(num):
Jason Pallone
895 PointsJason Pallone
895 PointsGotcha thanks a ton! Now so i completed the challenge but i'm slightly confused return not num % 2 if even_odd(num):
so that return means that if the number cant be divided by 2? then my other question was, how did running the if even_odd(num) test the number?
Steven Parker
241,809 PointsSteven Parker
241,809 PointsThe "even_odd" function returns a boolean (True/False) value, so you can test it directly.
The expression "not num % 2" is True if dividing num by 2 leaves a 0 remainder, and False otherwise.
Jason Pallone
895 PointsJason Pallone
895 Pointsok thank you for all the help, so if i did return num % 2, that means its true if it does not have a 0 remainder and false if it does? I just don't really understand how return works i guess
Steven Parker
241,809 PointsSteven Parker
241,809 PointsAll "return" does is pass back the value, the expression does all the work. By itself, "num % 2" is the remainder of dividing "num" by 2. So if the number is even, you get 0, and if it's odd, you get 1. But "not" converts that into a boolean (True/False) and then give the opposite value, so if the remainder was 0 you get True, and if it was 1 you get False.
So "
if even_odd(num):
" is the same thing as "if True:
" for even numbers and "if False:
" otherwise.Jason Pallone
895 PointsJason Pallone
895 PointsThank you very much for all the help!