Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial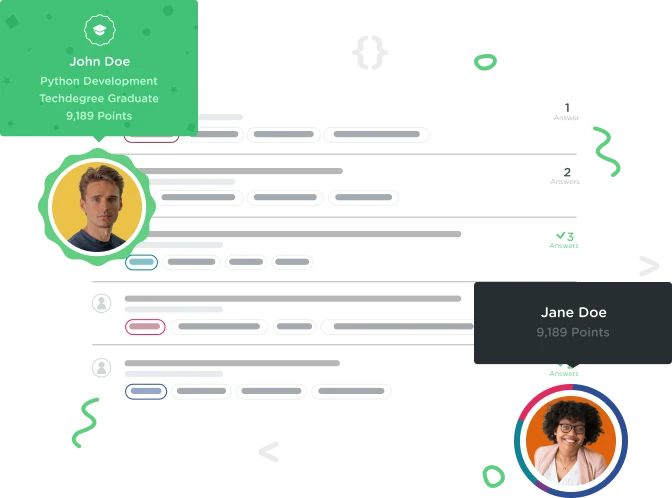

Anthony Cruz
Courses Plus Student 2,035 PointsEven when I guess right I am wrong?
Followed the coding with the video and it seems I followed Dave pretty well, But when I test the program and guess correctly (example) it tells me sorry the number was 5. Even if i guessed 5. Can someone tell me what I am missing.
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6 ) + 1;
var guess = prompt('I am thinking of a number between 1 and 6. What is it?');
if (parseInt(guess) === randomNumber ) {
correctGuess = true;
} else if (parseInt(guess) > randomNumber) {
var guessLess = prompt('Try Again. The number I am thinking of is less than '+ guess);
if (parseInt(guessLess) === randomNumber){
guessLess = true;
}
} else if (parseInt(guess) < randomNumber) {
var guessMore = prompt('Try Again. The number I am thinking of is More than ' + guess);
if (parseInt(guessMore) === randomNumber) {
guessMore = true;
}
}
if ( correctGuess ) {
document.write('<p>You guessed the number!</p>');
} else {
document.write('<p>Sorry. The number was ' + randomNumber + '.</p>');
}
2 Answers
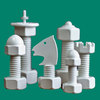
Steven Parker
231,533 PointsIt looks like you should get the correct result if you get it on the first guess. But on a second guess, the program sets either "guessLess" or "guessMore" instead of "correctGuess" so it will always be judged incorrect.
Fix the issue by changing each assignment to set "correctGuess".

Chris Troy
Courses Plus Student 7,395 PointsIn your else if() statements you have the boolean value being set to guessLess and guessMore. Try changing the variable correctGuess instead so it matches your ending if() statement.
Kyle Knapp
21,526 PointsKyle Knapp
21,526 PointsYour final conditional
if ( correctGuess ) {
only checks whether correctGuess is true. It doesn't check guessLess or guessMore. So even if they are set to true, your final conditional isn't testing them, it's only testing correctGuess, which is set to false at the top of your program.Since
if ( correctGuess ) {
is only testing correctGuess, it will always return false until correctGuess is re-set to true. So your sorry message will always print. (Unless you guess the number correct on your first try, which sets correctGuess here:if (parseInt(guess) === randomNumber ) { correctGuess = true;
and will causeif ( correctGuess ) {
to print your victory message.So, you either need to test for guessMore and guessLess in your final conditional:
if ( correctGuess || guessMore || guessLess)
or you need to change bothguessLess = true;
andguessMore = true;
tocorrectGuess = true
.In the first case, you are testing whether any of guessMore, guessLess or correctGuess is true, so if any one of them is true, even if the other two are false, the statement will evaluate to true and your victory message will print.
In the second case, you set correctGuess to true when either
parseInt(guessMore) === randomNumber
orparseInt(guessLess) === randomNumber)
. Since correctGuess is now set to true, your final conditional will pass and your victory message will print.