Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial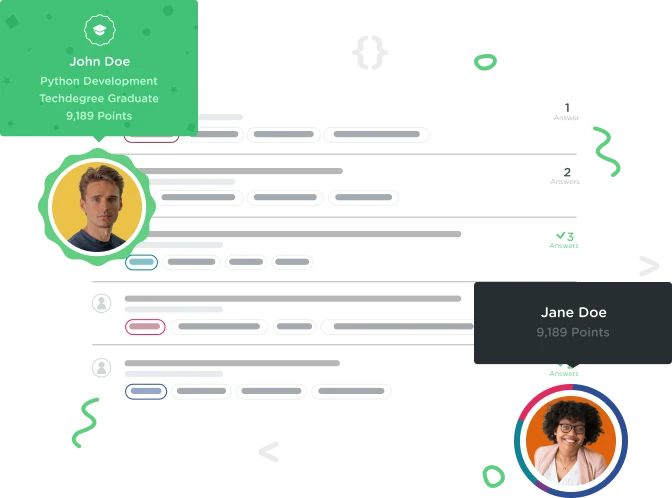

Nigel Chia
909 Pointseven_odd
code runs for forever
import random
def even_odd(num):
return num%2
number=random.randint(1,99)
while True:
if number == even_odd :
print('{} is even'.format(number))
break
else:
print('{} is odd'.format(number))
break
1 Answer

Ken Alger
Treehouse TeacherNigel;
Let's walk through your code a bit here to see why it has some problems running.
your_code.py
import random
def even_odd(num):
return num%2
number=random.randint(1,99)
while True:
if number == even_odd :
print('{} is even'.format(number))
break
else:
print('{} is odd'.format(number))
break
Your even_odd
function is taking a number and then just returning the value of the result of the remainder of "num divided by two", which is what that %
operator does. For example, if our number was five, even_odd
would be returning a value of one. While that is useful, that isn't really what the challenge is wanting, we'll get into the challenge more in a moment. I'd like to walk through more of your specific code first.
Let's next look at your While
statement. I can definitely see what you are trying to accomplish there, but there are some problems in there. First, you are comparing the randomly generated number
variable to, the way the current syntax is, an undefined variable, even_odd
. That should cause the script to stop with a NameError
.
Let's assume that we change that first comparison to use the even_odd
method by if number == even_odd(number)
. That's closer, right? Because we're now using the method and doing something to the number. However, think through what is actually going on in that While
statement.
Let's say, for example, that our random number is 62. We would expect to be told that it is even, right? If we walk through the code, we get if 62 == even_odd(62): ...
But what does even_odd(62)
return? It would return a value of zero, correct? Because 62%2
doesn't have a remainder. So our conditional check would put us into the "odd" else
statement.
Okay, now what about the code challenge itself?
We want our function to return True
if a number is even and False
if it is odd. Therefore all of our conditional checking needs to take place inside our function. Knowing that number % 2
will be zero if the number is even, we can use that in our conditional check, for example:
if number % 2 == 0:
return True
I'd like to see you work out the actual function on your own, Nigel, so I won't just give it all to you. :-) Hopefully, my wall of text here provides some explanation not only of where your code has been, but where it needs to go.
Also, for this challenge, you don't need to worry about generating numbers yourself. The challenge engine will generate random numbers and pass them into even_odd
when it checks your answer. You really only need to write a function that returns True
for even numbers and False
for odd numbers.
Post back if you're still stuck.
Ken