Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial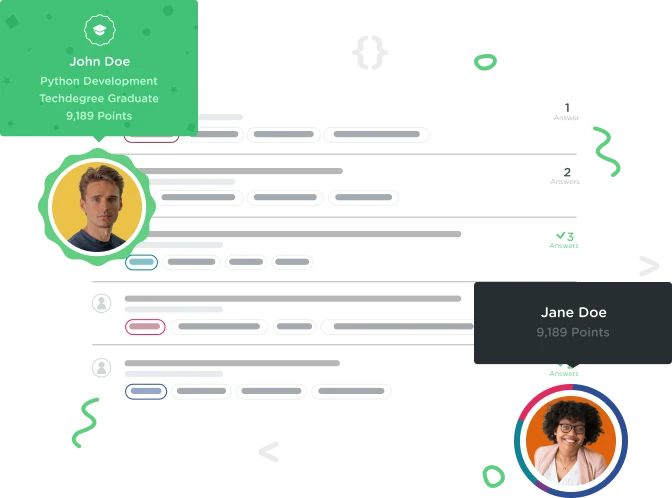

Ali Khan
5,229 Pointseven_odd challenge help please ("while" indentation)
I have a question regarding the indentation process in Python. I used workspaces to check my work for the even_odd challenge and found that indenting the "while" loop (including the "while start:") was giving me an indent error. I unindented it and it worked fine (see last code below). Here comes my problem:
In the letter_game refinement video Kenneth has two of the "while" loops indented after the "def get_guess():" and "def play():" functions:
def get_guess(bad_guesses, good_guesses):
while True:
# takes guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guesses, good_guesses)
Why does the code work here with the indentation and not in the even_odd challenge? Does it have to do with scopes? I would really appreciate it if someone could explain this to me so I don't keep guessing when to indent my code. (As you can see below the challenge doesn't let me pass unless I unindent the "while" loop.) Thank you.
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
num = random.randint(1,99)
if even_odd(num):
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
1 Answer
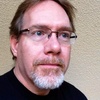
Chris Freeman
Treehouse Moderator 68,425 PointsThere are three basic parts to indentation.
- Ending a line with a colon (:). This signifies that a code block will start with the next statement
- The codeblock below the statement with a colon, must be indented a uniform amount. Can be anything but convention is 4 spaces.
- Any statement that has a different indentation from the current codebock must be a newly indented codeblock, or line up with a less-indented line from an earlier codeblock (this closed the current codeblock)
See comments in code:
import random
start = 5
def even_odd(num): # <-- Colon - start new codeblock
# If % 2 is 0, the number is even. # <-- first line of codeblock established indentation
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start: # <-- Error: This change in indentation does not match current or previous codeblock
num = random.randint(1,99)
if even_odd(num):
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
If you remove the SPACES before the while
so it aligns with the def
above. all should work
Ali Khan
5,229 PointsAli Khan
5,229 PointsThank you for your quick response Chris. I removed the spaces (unindented) from "while" and it did work. One more thing that I do not understand which I mentioned was how the letter_game refinement had the "while" loop uniformly indented (4 spaces) and it was working but that same uniform indentation applied to the even_odd game would result in an unexpected indent error(I aligned the "while start:" with even_odd(num)). Similarly removing the spaces (unindenting) from "while" in letter_game refinement and aligning it with the def get_guess(): would result in an indent error. Basically having the same format of indentation (4 spaces) or not having any spaces resulted in an error for the even_odd game and the letter_game respectively.
Chris Freeman
Treehouse Moderator 68,425 PointsChris Freeman
Treehouse Moderator 68,425 PointsThe difference between
letter_game
and the use here is where thewhile
loop is needed.In
letter_game
thewhile
loop is part of the function definitions so it's indented the extra 4 spaces. If the twowhile
loops were indented to match thedef
then while loops would not be a part of this functions.In this challenge, the
while
loop is not a part ofeven_odd
so it is not indented the extra 4 spaces.Does this make sense?
Ali Khan
5,229 PointsAli Khan
5,229 PointsYes, it does make sense now. After going through the examples again and reading your response alongside I got it. Thanks a lot! Really appreciate it.