Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial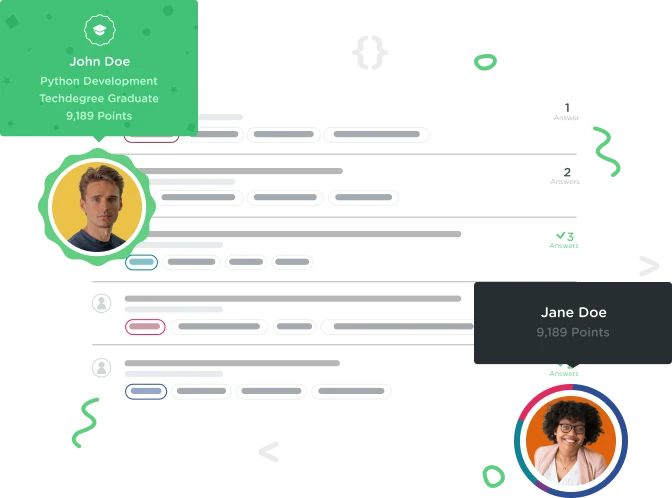

Sam Bui
878 Pointseven_odd challenge issue
Hi, everyone, I am doing even_odd chanllenge. At first, everything work just fine until the last step. I do everything the question asks for, but it still doesn't work. I know it has to be something with the line 'return not num % 2'. But that also the part I don't understand
If they only ask to pick a random number from 1 to 99 and see whether it is even or odd in 5 turns, what would they need the return statement anyway, because everything is done in even_odd function and the result is printed out straight away, why they need to store the value that 'not num % 2'. Why we need to do that.
In fact, to check whether the 'return not num % 2' has any effect on the code. I test my cod in PyCharm, the code I test doesn't have the return statement and it works beautifully
import random
def even_odd(num): # If % 2 is 0, the number is even. if num % 2 == 0: print('{} is even.'.format(num)) # Since 0 is falsey, we have to invert it with not. else: print('{} is odd.'.format(num))
start = 5
def even_odd_game(): start = 5 while start != 0: num = random.randint(1, 99) even_odd(num) start -= 1
even_odd_game()
So can someone explain for me why they include the return statement, and what I can do about it.
Thank you
# HERE IS WHAT THEY GIVE
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
# HERE IS MY ACTUAL CODE
import random
def even_odd(num):
# If % 2 is 0, the number is even.
if num % 2 == 0:
print('{} is even.'.format(num))
# Since 0 is falsey, we have to invert it with not.
else:
print('{} is odd.'.format(num))
return not num % 2
start = 5
while start != 0:
num = random.randint(1, 99)
even_odd(num)
start -= 1
2 Answers

Mike Wagner
23,559 PointsThe return statement exists in even_odd
to return the result (in this case a True
or False
) of the operation back to the function call (your even_odd(num)
). Essentially, you should leave the even_odd()
function alone and write all of your code inside the while
loop, though it can be accomplished in any number of other ways. Since the Challenge is expecting it to be done with the even_odd()
function left untouched, we'll approach it that way.
By moving your code
if num % 2 == 0:
print('{} is even.'.format(num))
else:
print('{} is odd.'.format(num))
down into the while statement below your call to even_odd()
and changing the num % 2 == 0
portion to be your function call like so:
start = 5
while start != 0:
num = random.randint(1, 99)
if even_odd(num):
print('{} is even'.format(num))
else:
print('{} is odd'.format(num))
start -= 1
You'll achieve the results you need.
*** Edit *** I missed that you had an extra "." in your print strings, so it wasn't passing because of that as well. If you remove them like above, you'll be golden.

Sam Bui
878 PointsOh, I think i get it now, so they mean that when num % 2 is 0, the even_odd(num) becomes true and it will trigger if even_odd(num): to print out '{} is even'
if num % 2 not equal 0, then even_odd(num) is false, in this case is 'else:' it will then say '{} is odd'
that makes sense, thank you for your help

Mike Wagner
23,559 PointsSam Bui - Yes, the way the even_odd()
function is set up, num % 2
will return
either 1
(a truthy value) or 0
(a falsy value). Since we actually need the opposite results we return
the not
version of those results, so True
becomes False
and vice versa. My modification to your code basically says "hey, this number is even" and the even_odd()
function is like "True!" unless we pass it an odd and it will be like "nah, that's not right." Then we just print off based on that response.