Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial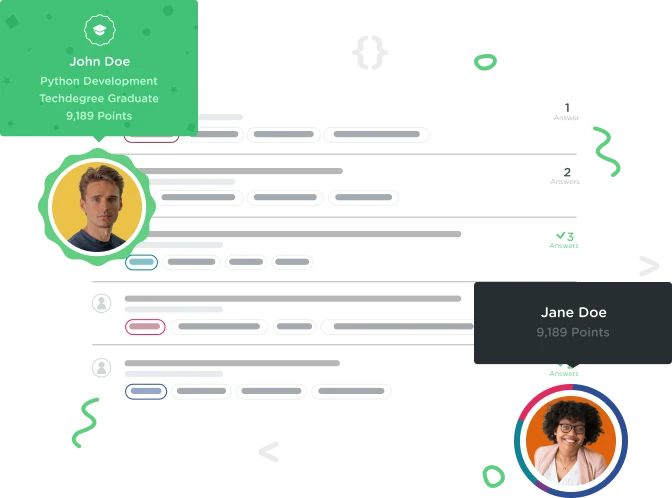

Idris Abdulwahab
Courses Plus Student 2,961 PointsEven_Odd Loop
My attempt to solve a code challenge is not panning through. Please help!
Code Challenge:
Treehouse starter code provided:
def even_odd(num): # If % 2 is 0, the number is even. # Since 0 is falsey, we have to invert it with not. return not num % 2
Code challenge tasks:
First task: For this challenge, we're going to need to use the random library. Add an import to the top of your file to bring it in.
Second task: Here's another easy step. Make a new variable, outside of the even_odd function, named start that's assigned to the number 5.
Third task: Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1.
import random
start = 5
while start:
even_odd(start)
start -= 1
def even_odd(num):
num = random.randint(1, 99)
# If % 2 is 0, the number is even.
if num % 2:
print("{} is even".format(num))
else:
# Since 0 is falsey, we have to invert it with not.
return not num % 2
print("{} is odd".format(num))
even_odd(num)
1 Answer

Krishna Pratap Chouhan
15,203 PointsFirstly you dont have to write even_odd function yourself. The starter code given is sufficient to implement the functionality and no additional code is needed there.
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
The above code will return opposite of expression 'num%2'.
you have to import random which will be used to get the radom-number-generator.
import random
And create a variable 'start' with value 5.
start = 5
finally write a while loop that loop through the non-zero value of start variable.
while start:
#some code here.
Inside the loop:
- get a random number between 1 and 99 using random.randint(1,99)
- print if the number is even or odd using format function
#random number
num = random.randint(1,99)
#checking if number is even or odd
if even_odd(num):
#printing the number as per the result.
print('{} is odd/even'.format(num))
Final code:
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
import random
start = 5
while start:
num = random.randint(1,99)
if even_odd(num):
print('{} is even'.format(num))
else:
print('{} is odd'.format(num))
start -= 1
Idris Abdulwahab
Courses Plus Student 2,961 PointsIdris Abdulwahab
Courses Plus Student 2,961 PointsHi Krishna. Thank you for your help. However I need clarification on the function itself. There was no code that carried out operation of dividing the number by 2. Or is that done by the "return not num % 2" ? I haven't studied the documentation up to that point. More clarifications please. Thank you.
Krishna Pratap Chouhan
15,203 PointsKrishna Pratap Chouhan
15,203 Pointsin a programming language, this is something a function does... broadly.
input -> [function] -> output
Now function returns the output using 'return' keyword.
But you know that already.
So, lets talk about the line
return not num%2
everything after return is an expression(tokens+operators).
Now if there is some problem in the way these tokens(or literals) and operators are arranged we get an error(most probably a syntax error).
Wait... you know that too.
In our expression we already have identified two operators... 'not' and '%'. Lets act like a compiler and start Expression evaluation:
Hope it helped, if not please do mention so.