Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial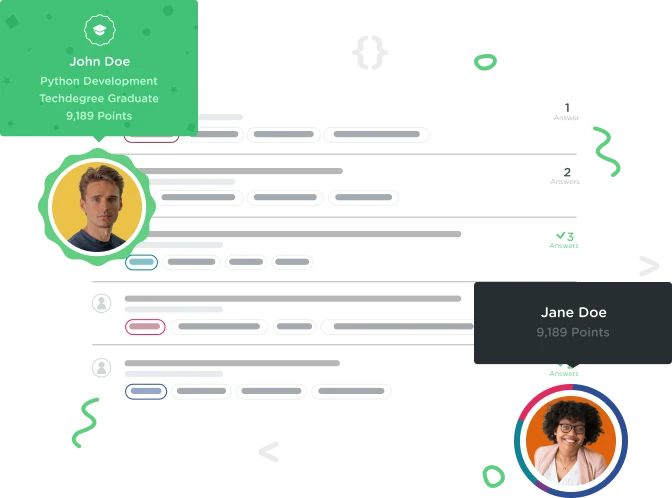

Robbie Bagley
2,189 Pointseven.py
Hello I'm still having a problem solving this. Here is the original problem. I know I shouldn't alter the odd_else but just call it but I I'm not sure where I'm making a mistake. I would appreciate any help. Thank you!
Alright, last step but it's a big one. Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1. I know it's a lot, but I know you can do it!
import random
start = 5
def even_odd(num):
while start != None:
num = random.randint(1,99)
if num % 2 == 0:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
size -= 1
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
1 Answer
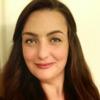
Jennifer Nordell
Treehouse TeacherHi there! I see you're still working on this, and you're right, you're not supposed to alter the even_odd
function. So let's break down the instructions a bit.
At the end of step 2 you should have had something much like this:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
Now the next step of the instructions says "Make a while loop that runs while start is falsey." The even_odd
function is already coded and provided to you. You are not meant to alter it in any way. It will return true if the number is even, and false if the number is odd.
So to make a while loop that runs while start is "truthy", we can do this:
while(start):
This tells the loop to run while start is "truthy". You are creating an endless loop. You say to run the loop while start
is not equal to None
, but there is nothing in your code that even has the possibility of changing the value of start to None
. The value of start
begins at 5. It will become "falsey" when it reaches 0. So really, we just want to count down from 5 to 0 and stop when it's 0.
Now you already have the even_odd
function to tell you if a number is even or odd so this comparison is unnecessary:
if num % 2 == 0:
Instead, we want to send in that number to our already coded function. Also, you're trying to decrement the value of a variable named size
, but that variable doesn't exist. Let's take a look at the completed code:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while(start): # while the value of start is "truthy" but not necessarily equal to True
num = random.randint(1,99) #get a random number between 1 and 99
if even_odd(num): # if the result returned from the even odd function was true
print("{} is even".format(num)) #print that the number was even
else: #otherwise
print("{} is odd".format(num)) #print that the number was odd
start -= 1 #decrement start
I hope this helps, but let me know if you have any questions!
Robbie Bagley
2,189 PointsRobbie Bagley
2,189 PointsIt worked! Thank you so much!