Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial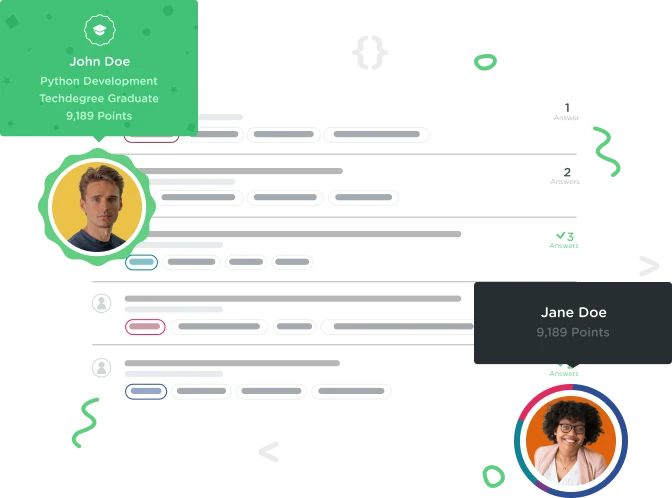
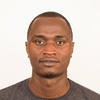
Dominic Motuka
5,124 PointsEvent Bubbling vs Event Propagation vs Event Capturing
I find the concept of event bubbling
a little cryptic.
Just so when I thought I had grasped the concept, MDN told me otherwise.
Done a little research on it and I seem to come up with more questions:
- What if the elements have different listeners
- How are the events propagated/ captured: which direction ( can I assume itβs inwards ==>> up only )
- What is the event target? Does it have to be the innermost element?
1 Answer

Tomas Svojanovsky
26,680 Points3. Assume I clicked on three element. event.target is element where event occurred and the opposite of this is currentTarget - element where event was attached.
<ul class="list">
<li class="one">One</li>
<li class="two">Two</li>
<li class="three">Three</li>
</ul>
<script>
document.querySelector('.list').addEventListener('click', (e) => {
const target = e.target;
const currentTarget = e.currentTarget;
console.log(target, 1); // three
console.log(currentTarget); // list
});
</script>
2. You can control the direction of bubbling. addEventListener had third param to achieve this. Assume the same html as previous example. If you change third param to true or false and then click on element .one you will get different results.
document.querySelector('.list').addEventListener('click', (e) => {
const target = e.target;
const currentTarget = e.currentTarget;
console.log(target, 1);
console.log(currentTarget);
}, true);
document.querySelector('.one').addEventListener('click', (e) => {
const target = e.target;
const currentTarget = e.currentTarget;
console.log(target, 2);
console.log(currentTarget)
});
- It's probably related to the previous example. If you don't change useCapture param they will be called in the order you register them. If you change bubbling direction (lookup) it depends on your code and html structure.