Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial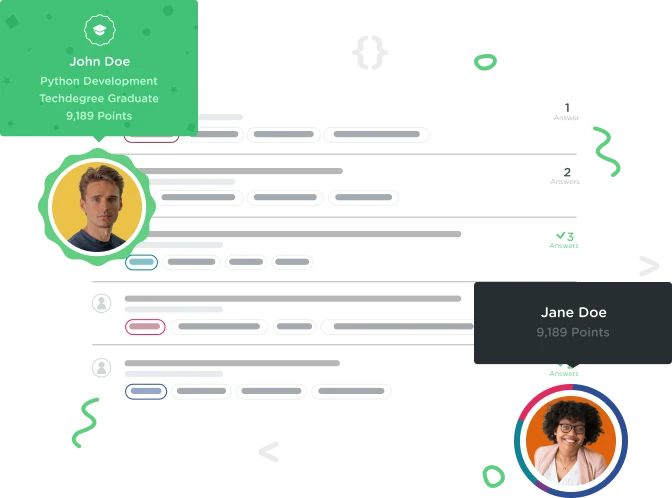
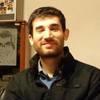
Axel Perossa
13,930 Pointsevent handler binding
I see that one approach to binding event handlers in React is doing it in the constructor. As per the docs:
class Foo extends Component {
constructor() {
super();
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log('Click happened');
}
}
My question is how can we call the bind function on this.handleClick before the method is even defined? I believe the constructor is the first code to run when a new instance of Foo is created, so why isn't this.handleClick undefined
this.handleClick = this.handleClick.bind(this);
if handleClick() is defined below that? Some kind of hoisting?
1 Answer
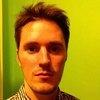
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsI'm going to tentatively answer this, because I'm not 100% sure. But I think the reason it works is that handleClick
gets defined on the prototype, so then it's there when you create an instance later. It's not hoisting.
Side note, arrow functions make this so much better. You can assign an arrow function to a class property instead of using a class method, and then you don't have call .bind() on it in order to pass it down as an event handler. (This is covered in other videos/courses)