Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial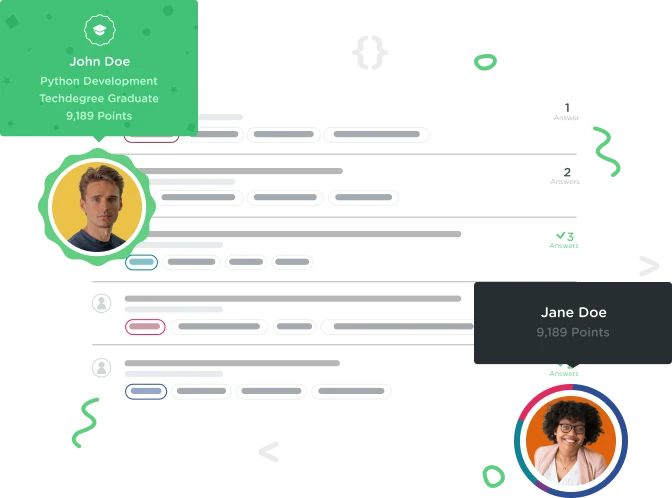
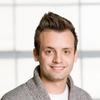
David Loder
4,987 PointsEvent listener button won't go away after clicking it
So after following the lesson, I tested the code to see that it works but the button doesn't disappear. Any thoughts?
1 Answer

KRIS NIKOLAISEN
54,971 PointsCheck the console. You have an error here:
<img src=${person.thumbnail.source}>
This is due to ambiguity with the names of two astronauts not retrieving the correct page: Alexander Skvortsov and Andrew Morgan.
I modified getPeopleInSpace()
to use the disambiguated pages by using Aleksandr_Skvortsov_(cosmonaut) and Andrew_R._Morgan respectively.
// Handle all fetch requests
async function getPeopleInSpace(url) {
const peopleResponse = await fetch(url);
const peopleJSON = await peopleResponse.json();
const profiles = peopleJSON.people.map( async (person) => {
const craft = person.craft;
var fetchurl = wikiUrl + person.name
if (person.name == "Alexander Skvortsov") {
fetchurl = "https://en.wikipedia.org/api/rest_v1/page/summary/Aleksandr_Skvortsov_(cosmonaut)"
}
if (person.name == "Andrew Morgan") {
fetchurl = "https://en.wikipedia.org/api/rest_v1/page/summary/Andrew_R._Morgan"
}
const profileResponse = await fetch(fetchurl);
const profileJSON = await profileResponse.json();
return { ...profileJSON, craft };
});
return Promise.all(profiles);
}
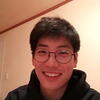
Seokhyun Wie
Full Stack JavaScript Techdegree Graduate 21,606 PointsKRIS NIKOLAISEN Hi Kris, thanks for the answer. Just to understand it more clearly, can you explain why it does not disappear in a structural way? What I mean is that before we put async and await, the event.target(=button).remove() worked no matter what error occurs in the middle. Thanks in advance.
David Loder
4,987 PointsDavid Loder
4,987 Points