Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial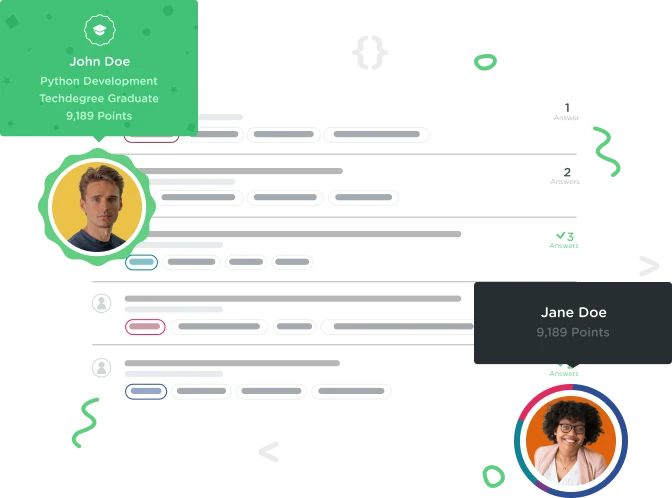
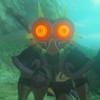
tomasvukasovic
24,022 PointsEvents Deprecated, This video needs to be updated
So, Andrew on the profile JS file imports events, and uses EventEmitter which has been deprecated, also as I putted previously the profiles have been moved to https protocol. I guess It would be best to remove the whole course by now as it generates more doubts than answers.
I will try to make the profile JS again so it works with the course and upload it as an answer to this thread.
3 Answers
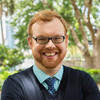
Andrew Chalkley
Treehouse Guest Teachervar EventEmitter = require("events").EventEmitter;
var https = require("https");
var http = require("http");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
var profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
The profile.js file has already been updated. If you've taken the course previously you'll have the older version in your workspace.
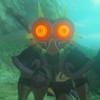
tomasvukasovic
24,022 PointsKangin Choi yes! https://nodejs.org/api/events.html#events_class_eventemitter for example at the listenerCounter method it suggests that we use the emitter.listenerCount() instead. This post is from a while back and I had this issue because I was using a older version of the project, the current version has everything updated and there are no deprecations. Still a good practice is to read the docs and check if something you may be using is now deprecated, and analyze why it was deprecated and what is the newer solution. So don't worry and happy codeing !
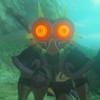
tomasvukasovic
24,022 PointsHey Kangin Choi EventEmitter was a class from nodeJs that has some methods now deprecated, it gave us the possibility to add new listeners, remove them, count them, etc. The documentation has all the info you need

Kangin Choi
4,174 PointsIf it is deprecated, then is there any other replacement I should be familiar with?
Kangin Choi
4,174 PointsKangin Choi
4,174 PointsCan you please explain what EventEmitter does?