Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial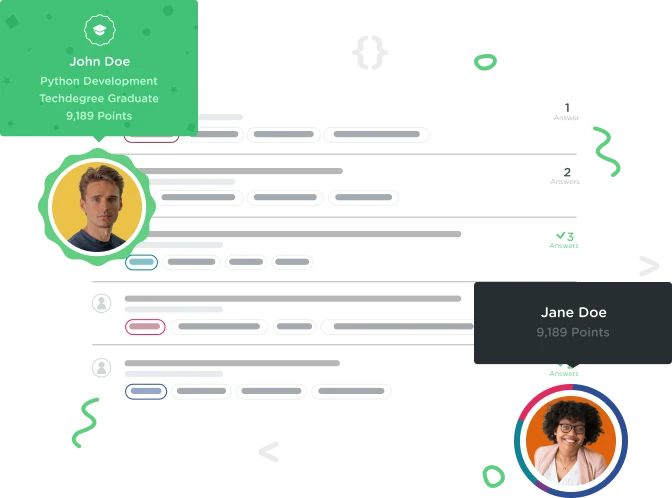
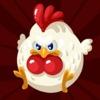
Binyamin Friedman
14,615 PointsEvery hourly list item is the same!
Every list item is the same and I have no idea why.
Hour.java
package com.teamtreehouse.stormy.weather;
import android.os.Parcel;
import android.os.Parcelable;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Hour implements Parcelable {
private long mTime;
private String mSummary;
private double mTemperature;
private String mIcon;
private String mTimezone;
public Hour() { }
public Long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public int getTemperature() {
return (int) Math.round(mTemperature);
}
public void setTemperature(double temperature) {
mTemperature = temperature;
}
public String getIcon() {
return mIcon;
}
public int getIconId() {
return Forecast.getIconId(mIcon);
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public String getHour() {
SimpleDateFormat formatter = new SimpleDateFormat("h a");
Date date = new Date(mTime * 1000);
return formatter.format(date);
}
@Override
public int describeContents() {
return 0; // ignore
}
@Override
public void writeToParcel(Parcel parcel, int i) {
parcel.writeLong(mTime);
parcel.writeString(mSummary);
parcel.writeString(mIcon);
parcel.writeDouble(mTemperature);
parcel.writeString(mTimezone);
}
private Hour(Parcel in) {
mTime = in.readLong();
mSummary = in.readString();
mIcon = in.readString();
mTemperature = in.readDouble();
mTimezone = in.readString();
}
public static final Creator<Hour> CREATOR = new Creator<Hour>() {
@Override
public Hour createFromParcel(Parcel parcel) {
return new Hour(parcel);
}
@Override
public Hour[] newArray(int i) {
return new Hour[i];
}
};
}
HourAdapter.java
package com.teamtreehouse.stormy.adapters;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.teamtreehouse.stormy.R;
import com.teamtreehouse.stormy.weather.Hour;
public class HourAdapter extends RecyclerView.Adapter<HourAdapter.HourViewHolder> {
private Hour[] mHours;
public HourAdapter(Hour[] hours) {
mHours = hours;
}
@Override
public HourViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.hourly_list_item, parent, false);
HourViewHolder viewHolder = new HourViewHolder(view);
return viewHolder;
}
@Override
public void onBindViewHolder(HourViewHolder holder, int position) {
holder.bindHour(mHours[position]);
}
@Override
public int getItemCount() {
return mHours.length;
}
public class HourViewHolder extends RecyclerView.ViewHolder {
public TextView mTimeLabel;
public TextView mSummaryLabel;
public TextView mTemperatureLabel;
public ImageView mIconImageView;
public HourViewHolder(View itemView) {
super(itemView);
mTimeLabel = (TextView) itemView.findViewById(R.id.timeLabel);
mSummaryLabel = (TextView) itemView.findViewById(R.id.summaryLabel);
mTemperatureLabel = (TextView) itemView.findViewById(R.id.temperatureLabel);
mIconImageView = (ImageView) itemView.findViewById(R.id.iconImageView);
}
public void bindHour(Hour hour) {
mTimeLabel.setText(hour.getHour());
mSummaryLabel.setText(hour.getSummary());
mTemperatureLabel.setText(hour.getTemperature() + "");
mIconImageView.setImageResource(hour.getIconId());
}
}
}
HourlyForecastActivity.java
package com.teamtreehouse.stormy.ui;
import android.content.Intent;
import android.os.Parcelable;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import com.teamtreehouse.stormy.R;
import com.teamtreehouse.stormy.adapters.HourAdapter;
import com.teamtreehouse.stormy.weather.Hour;
import java.util.Arrays;
import butterknife.ButterKnife;
import butterknife.InjectView;
public class HourlyForecastActivity extends AppCompatActivity {
private Hour[] mHours;
@InjectView(R.id.recyclerView) RecyclerView mRecyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_hourly_forecast);
ButterKnife.inject(this);
Intent intent = getIntent();
Parcelable[] parcelables = intent.getParcelableArrayExtra(MainActivity.HOURLY_FORECAST);
mHours = Arrays.copyOf(parcelables, parcelables.length, Hour[].class);
HourAdapter hourAdapter = new HourAdapter(mHours);
mRecyclerView.setAdapter(hourAdapter);
RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(this);
mRecyclerView.setLayoutManager(layoutManager);
// If fixed size improve performance
mRecyclerView.setHasFixedSize(true);
}
}
MainActivity.java Note: there is some commented out code that is my kinda failed attempt to implement google play services for the location
package com.teamtreehouse.stormy.ui;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.drawable.Drawable;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.support.v4.content.res.ResourcesCompat;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.ImageView;
import android.widget.ProgressBar;
import android.widget.TextView;
import android.widget.Toast;
import com.teamtreehouse.stormy.R;
import com.teamtreehouse.stormy.weather.Current;
import com.teamtreehouse.stormy.weather.Day;
import com.teamtreehouse.stormy.weather.Forecast;
import com.teamtreehouse.stormy.weather.Hour;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import butterknife.ButterKnife;
import butterknife.InjectView;
import butterknife.OnClick;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
public static final String DAILY_FORECAST = "DAILY_FORECAST";
public static final String HOURLY_FORECAST = "HOURLY_FORECAST";
//public static final int PERMISSION_REQUEST_CODE = 0;
private Forecast mForecast;
//private FusedLocationProviderClient mFusedLocationProviderClient;
@InjectView(R.id.timeLabel) TextView mTimeLabel;
@InjectView(R.id.temperatureLabel) TextView mTemperatureLabel;
@InjectView(R.id.humidityValue) TextView mHumidityValue;
@InjectView(R.id.precipValue) TextView mPrecipValue;
@InjectView(R.id.summaryLabel) TextView mSummaryLabel;
@InjectView(R.id.iconImageView) ImageView mIconImageView;
@InjectView(R.id.refreshImageView) ImageView mRefreshImageView;
@InjectView(R.id.progressBar) ProgressBar mProgressBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.inject(this);
//mFusedLocationProviderClient = LocationServices.getFusedLocationProviderClient(this);
mProgressBar.setVisibility(View.INVISIBLE);
mRefreshImageView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
getForecast();
}
});
getForecast();
Log.d(TAG, "Main UI code is running!");
}
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) {
if (grantResults[0] == PackageManager.PERMISSION_DENIED || permissions.length == 0) {
Toast.makeText(this, "Stormy requires your location to function. Stormy won't ask for location permissions again, but you can always enable the permission in settings", Toast.LENGTH_LONG).show();
Toast.makeText(this, "Stormy requires your location to function. Stormy won't ask for location permissions again, but you can always enable the permission in settings", Toast.LENGTH_LONG).show();
Toast.makeText(this, "Stormy requires your location to function. Stormy won't ask for location permissions again, but you can always enable the permission in settings", Toast.LENGTH_LONG).show();
}
}
private void getForecast() {
toggleRefresh();
/*Location location = null;
int permissionCheck = ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION);
if (permissionCheck == PackageManager.PERMISSION_GRANTED) {
Task task = mFusedLocationProviderClient.getLastLocation();
location = (Location) task.getResult();
} else {
requestPermissions(new String[]{Manifest.permission.ACCESS_COARSE_LOCATION}, PERMISSION_REQUEST_CODE);
}*/
//if (location != null) {
String apiKey = "bb8d7f69ca1b7c515db4409ff9c0a97c";
//double latitude = location.getLatitude();
//double longitude = location.getLongitude();
double latitude = 0;
double longitude = 0;
String forecastUrl = "https://api.darksky.net/forecast/" +
apiKey + "/" + latitude + "," + longitude;
if (isNetWorkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
alertUserAboutError();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
runOnUiThread(new Runnable() {
@Override
public void run() {
toggleRefresh();
}
});
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mForecast = parseForecastDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUserAboutError();
}
} catch (IOException | JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
} else {
Toast.makeText(this, R.string.network_unavailable_message, Toast.LENGTH_LONG).show();
}
//}
}
private void toggleRefresh() {
if (mProgressBar.getVisibility() == View.INVISIBLE) {
mProgressBar.setVisibility(View.VISIBLE);
mRefreshImageView.setVisibility(View.INVISIBLE);
} else {
mProgressBar.setVisibility(View.INVISIBLE);
mRefreshImageView.setVisibility(View.VISIBLE);
}
}
//TODO one day add something that displays your location
private void updateDisplay() {
Current current = mForecast.getCurrent();
mTemperatureLabel.setText(current.getTemperature() + "");
mTimeLabel.setText("At " + current.getFormattedTime() + " it will be");
mHumidityValue.setText(current.getHumidity() + "");
mPrecipValue.setText(current.getPrecipChance() + "%");
mSummaryLabel.setText(current.getSummary());
Drawable drawable = ResourcesCompat.getDrawable(getResources(), current.getIconId(), null);
mIconImageView.setImageDrawable(drawable);
}
private Forecast parseForecastDetails(String jsonData) throws JSONException {
Forecast forecast = new Forecast();
forecast.setCurrent(getCurrentDetails(jsonData));
forecast.setHourlyForecast(getHourlyForecast(jsonData));
forecast.setDailyForecast(getDailyForecast(jsonData));
return forecast;
}
private Day[] getDailyForecast(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject daily = forecast.getJSONObject("daily");
JSONArray data = daily.getJSONArray("data");
Day[] days = new Day[data.length()];
for (int i = 0; i < data.length(); i++) {
JSONObject jsonDay = data.getJSONObject(i);
Day day = new Day();
day.setSummary(jsonDay.getString("summary"));
day.setIcon(jsonDay.getString("icon"));
day.setTemperatureMax(jsonDay.getDouble("temperatureMax"));
day.setTime(jsonDay.getLong("time"));
day.setTimezone(timezone);
days[i] = day;
}
return days;
}
private Hour[] getHourlyForecast(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject hourly = forecast.getJSONObject("hourly");
JSONArray data = hourly.getJSONArray("data");
Hour[] hours = new Hour[data.length()];
for (int i = 0; i < data.length(); i++) {
JSONObject jsonHour = data.getJSONObject(0);
Hour hour = new Hour();
hour.setSummary(jsonHour.getString("summary"));
hour.setTemperature(jsonHour.getDouble("temperature"));
hour.setIcon(jsonHour.getString("icon"));
hour.setTime(jsonHour.getLong("time"));
hour.setTimezone(timezone);
hours[i] = hour;
}
return hours;
}
private Current getCurrentDetails(String jsonData) throws JSONException {
Current current = new Current();
JSONObject forecast = new JSONObject(jsonData);
current.setTimeZone(forecast.getString("timezone"));
JSONObject currently = forecast.getJSONObject("currently");
current.setHumidity(currently.getDouble("humidity"));
current.setTime(currently.getLong("time"));
current.setIcon(currently.getString("icon"));
current.setPrecipChance(currently.getDouble("precipProbability"));
current.setSummary(currently.getString("summary"));
current.setTemperature(currently.getDouble("temperature"));
return current;
}
private boolean isNetWorkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
@OnClick (R.id.dailyButton)
public void startDailyActivity(View view) {
Intent intent = new Intent(this, DailyForecastActivity.class);
intent.putExtra(DAILY_FORECAST, mForecast.getDailyForecast());
startActivity(intent);
}
@OnClick (R.id.hourlyButton)
public void startHourlyActivity(View view) {
Intent intent = new Intent(this, HourlyForecastActivity.class);
intent.putExtra(HOURLY_FORECAST, mForecast.getHourlyForecast());
startActivity(intent);
}
}
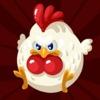
Binyamin Friedman
14,615 PointsI doubt it, but ok.
1 Answer
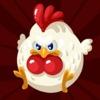
Binyamin Friedman
14,615 PointsWait, it actually was in MainActivity! I wasn't using the incrementing i variable for getting the hours in getHourlyForecast()
Thanks! (Even though it was kind of indirect help)
Calin Bogdan
14,921 PointsCalin Bogdan
14,921 PointsHello!
Do you mind adding the MainActivity.java file too? That way we might find the issue easier (or maybe the issue is actually there).
Thanks!