Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial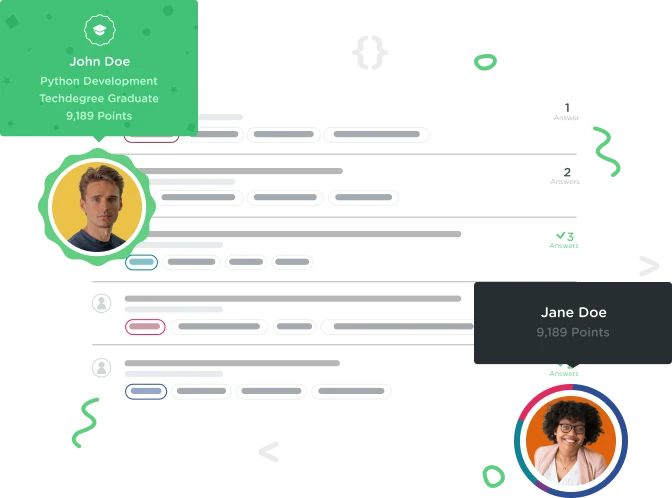

Lukasz Walczak
6,620 PointsEverything in Java is passed by value, is it not? :)
So how come original string was modified when passed to the method?
1 Answer
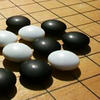
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsA very good question!
This is always confusing to beginners. Java is passed by value. But the interesting question is, what is the value of the variable?
- If the variable is a primitive data type (int, long, char, boolean, etc.) the variable contains the actual numeric value of the data you stored inside it.
- If the variable has an object as a data type, the value of the variable is a reference to the location of the object.
So let's say you have:
int number = 2;
public int sum(int i) {
return i + 1;
}
square(number);
number will still be 2. Your result will be stored in a new variable but it doesn't affect number.
Now with an object (let's say an array :-))
String[] colors = {"red", "blue", "green"};
public void replaceFirst(String[] array) {
array[0] = "yellow";
}
replaceFirst(colors);
In this case the colors array will now be {"yellow", "blue", "green"} Here, the "colors" value is a reference to an array object. That reference is like an address of the array object inside the memory. This reference is copied when the variable is passed to the method, and so the "array" variable inside the method points to the same object as "colors". It doesn't make a copy of the array, it makes a copy of the address to the array. So you can make changes to that original array from within the method.
But it's still not equivalent to "pass by reference". What you can't do is change the "colors" variable itself by making it point to some other object. For example this:
String[] colors = {"red", "blue", "green"};
public void setToNewArray(String[] array) {
array= new String[3];
}
setToNewArray(colors);
This will not set the "colors" variable to a new array! Because that would mean changing the value of the variable "colors", and making it point to a new object located elsewhere in the memory. The method can't change the "colors" variable itself.
I hope my explanations weren't too confusing!
Lukasz Walczak
6,620 PointsLukasz Walczak
6,620 PointsMany thanks for taking the time to answer! It is highly appreciated!
It is clearer and clearer. Slowly but surely, I'll get there eventually ;)