Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial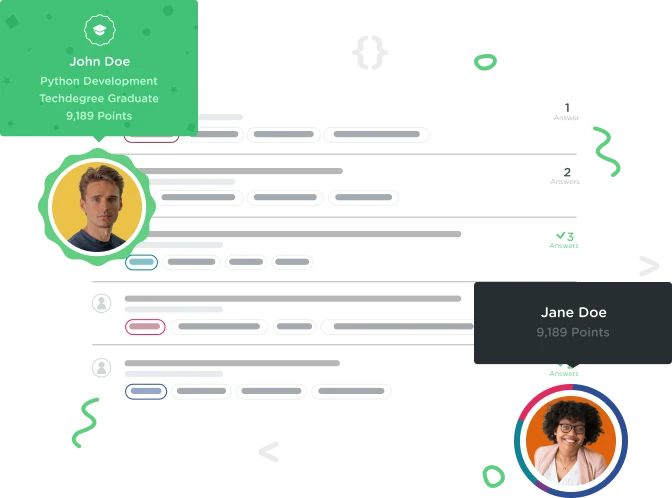

Tarun Jha
2,844 PointsExcept not working as it should!
import random
cmp_choice = random.randint(1,10)
def win_msg(): print('You win!') return
def lose_msg(): print('Sorry! you lost') return
def compare():
print('Type any integer b/w 1 to 10')
for chances in range(5):
try:
user_choice = int(input('What\'s your guess? '))
except ValueError:
print('{} is not an integer'.format(user_choice))
else:
if cmp_choice == user_choice:
win_msg()
break
else:
if user_choice > cmp_choice:
print('Nooo!,it\'s too much, my number is smaller than {}'.format(user_choice))
elif user_choice < cmp_choice :
print('You are too low, mine number is higher than {}'.format(user_choice))
print('Chances left: ',5 - chances)
else:
print('You didn\'t get it it was {}'.format(user_choice))
try_again = input('Wanna play again? ').upper()
if try_again == 'Y':
compare()
compare()
unboundLocalError at line 24
2 Answers

Ryan S
27,276 PointsHi Tarun,
An UnboundLocalError in Python gets raised when you try to use a variable that hasn't been assigned a value.
This is happening in your code because you are trying to convert a user input to an int and assign it to a variable all in one statement (in your try block).
Let's say you enter a non-numeric string. It will throw a value error like you'd expect because it can't be converted to an int. But the input value won't actually get assigned to "user_choice", because the error will be raised before that can happen.
Now when you get to your except block, you are trying to use "user_choice" in your string, but it has no value assigned to it yet, which gives you your UnboundLocalError.
To fix this, you can separate the try block into two steps. The first step would be get an input and assign it to "user_choice", then in the second step, try to convert it to an int. Or another option is to just remove the reference to "user_choice" in your string in the except block.
Hope this helps.

Tarun Jha
2,844 Pointsthank you
Krishna Pratap Chouhan
15,203 PointsKrishna Pratap Chouhan
15,203 PointsIf indentation is fine then i would say the line 24 has a function.
win_msg() is not shared. Could you please share it as well.