Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial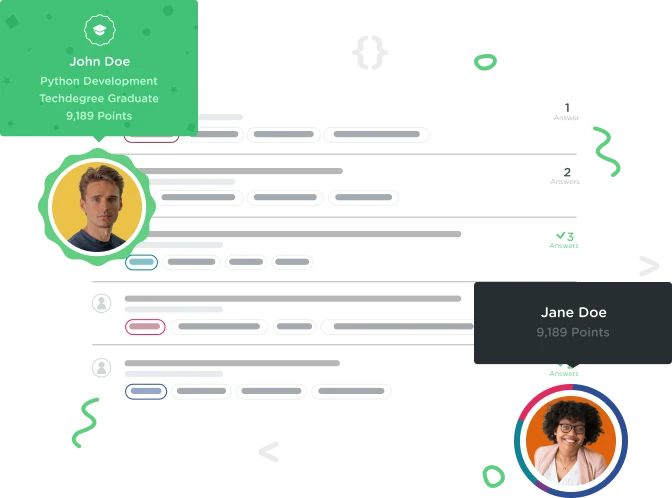
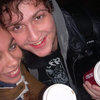
Bruno Correia
3,114 Pointsexcept ValueError as err
The solution to include our own error message makes sense when the issue is with the number of people, but, if the user replies to "What is the cost?" with "too much", this solution will make err be a "could not convert string to float" error, which is what the user will see. This does not seem very user-friendly and makes it look like the solution worked for the specific case in the video but would not be ideal in the real world.
Would there be a better solution to this problem to prevent this? Should we include another if in the function to check if the user did not enter a number on the "what is the total" question? How can we check that?
Thank you in advance for the help.
2 Answers
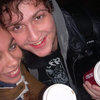
Bruno Correia
3,114 PointsKailash Seshadri thank you so much for the quick reply!
I found a way to make it work but am not sure if it's ideal. Here's how the script looks like now:
import math
def split_check(total, number_of_people):
if number_of_people <= 1:
raise ValueError("More than one person is required to split the check")
return math.ceil(total / number_of_people)
try:
total_due = float(input("What is the total? "))
except ValueError:
print("The total is not valid")
else:
try:
number_of_people = int(input("How many people? "))
amount_due = split_check(total_due, number_of_people)
except ValueError as err:
print("Oh no. That's not a valid value. Try again...")
print("({})".format(err))
else:
print("Each person owes ${}".format(amount_due))
This allows me to show "The total is not valid" if a ValueError is thrown, but changes the original script quite a bit. I also had to add a whole new try and except which maybe I don't even need. I've noticed that a similar problem happens if the number of people isn't an int. So if it's 0 or negative it shows the error message we've set up in the function. If it's e.g. a string it shows a not so user-friendly message about it being an invalid literal for int() with base 10
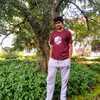
Kailash Seshadri
3,087 PointsThis is the soultion that I had in mind for when the user enters a non integer value for either number of people or a string. Great work! The idea of using the varibale err
is just sto show you that you can indeed do such a thing if you needed to. Maybe this program is for use only by other programmers :D? When youre designing an app for the general public, you would avoid prinitng out the error itself and instead just say "Please enter a valid number!".
You could also put this whole try block in a while loop so the program doesn't crash when the user enters an invalid number, but loops back to the trast and asks the question again and again until a valid reply is received. I did this on a hangman game I was working on a while back as a fun project, and a part of the code is below.
while True:
guess = input("guess a letter: ")
if len(guess) != 1:
print("You can only guess one letter at a time")
print()
continue
elif guess in letters_guessed:
print('You have already guessed', guess + '!')
print('Try again')
continue
else:
break
Enterring an invalid guess would just make the program ask for the guess again, and wouldnt leave the while loop until the user guesses a valid letter. So keep thinking like this, and working an different solutions for the problems that you see with any program you write. Some solutions may work, and some wont, but you'll learn a lot about syntax and how flexible functions are by just experimenting. When something doesn't work out, you can always hop on to the comminty here and drop a question if you ever get stuck, and someone will help you out. Good luck!
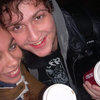
Bruno Correia
3,114 PointsThat sounds amazing, thank you very much for your help!
Kailash Seshadri
3,087 PointsKailash Seshadri
3,087 PointsTry make an attempt at a solution of your own and if you run into issues, feel free to reply to this and I'll help you out! Hint: You could use the try block in a different way!