Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial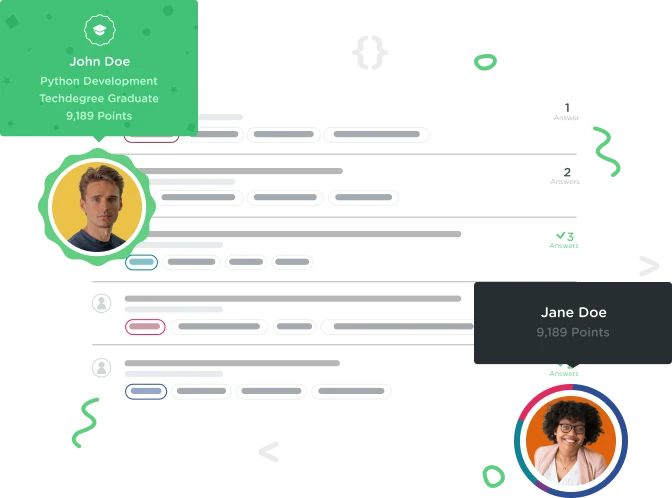
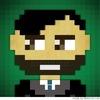
Tamas Gonda
7,332 Pointsexcept ValueError doesn't seems to work fine!
Hello Dear friends! I've done what Dave said. used try and then except finished off with an else. The program seems working fine, as it's throws the message that it's not a number, if I type anything else, BUT: it prints the last typed number instead of the letter. So for example I type 3 = try again, 6 = try again, H = 6 is not a number! if the first try is a letter, it gives me an error message: During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "number_game.py", line 17, in <module>
game()
File "number_game.py", line 9, in game
print("{} is not a number!".format(guess))
UnboundLocalError: local variable 'guess' referenced before assignment
while len(guesses) < 5:
try:
guess = int(input("Try guess my number!"))
except ValueError:
print("{} is not a number!".format(guess))
else:
3 Answers
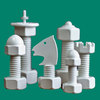
Steven Parker
231,275 PointsThe variable guess is only assigned when the int conversion is successful, so when the exception occurs, it still contains the last valid guess. Of course, if the very first input causes the exception, it has not been assigned yet at all.
You can resolve this by using separate steps for getting the input and converting it to a number:
while len(guesses) < 5:
answer = input("Try to guess my number!")
try:
guess = int(answer)
except ValueError:
print("{} is not a number!".format(answer))
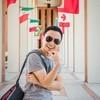
hamsternation
26,616 PointsHey actually, if you see the teacher's note, that is an error in the code.
Just throw out the .format part of the code and don't use the {} and you should be fine.
Teacher's Note:
Did you catch the possible problem where we take a user's guess during the game? Since we convert their input()'d guess into an int right away, if they give us something that can't be turned into a number, it'll throw a ValueError. So far, so good, 'cause that's what our try is for, right?
Except in the except ValueError: we used the guess variable, which will be undefined and throw it's own new error. Oh no! Just take out the string formatting for that error message, though, and you should be good to go.
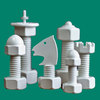
Steven Parker
231,275 PointsThat would fix the issue, but it gives up repeating the bad input in the error message. The solution I suggested resolves the issue and keeps that feature.
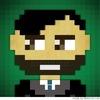
Tamas Gonda
7,332 PointsYeah, I totally missed that! My bad! Thank you!
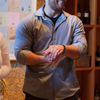
Jonathan Ankiewicz
17,901 Pointsif your running it locally you may need to change input to raw_input
Tamas Gonda
7,332 PointsTamas Gonda
7,332 PointsThank you so much! It was bothering me a lot! So just simply have to declare the variable earlier, and that holds the value, whatever it is.
Vanessa Van Gilder
7,034 PointsVanessa Van Gilder
7,034 PointsIs there a reason you made a new variable called answer? I did this and just kept it as guess.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsI made "answer" represent what was given by the user, and kept "guess" to represent a successfully converted number value.