Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial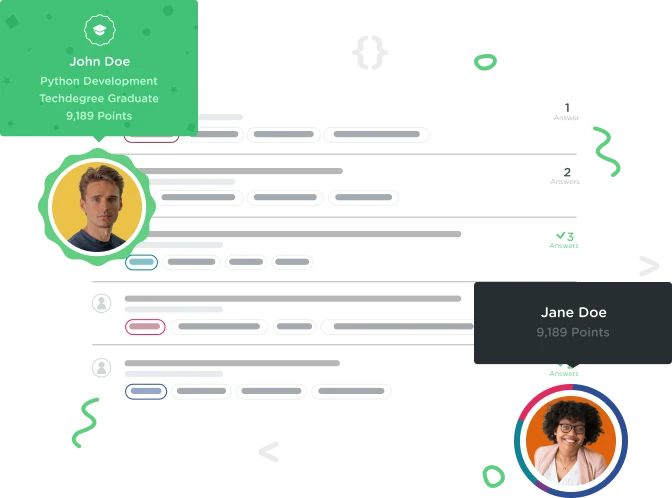

Tashfin Shahid
1,298 PointsException error (Java)
I don't understand what is wrong?
Challenge
// You probably already spotted the error in the drive method that accepts laps. You can drive way more laps than our battery can handle. Let's fix it.
Add logic to the drive method so that it throws an IllegalArgumentException if there aren't enough bars to support the laps request. Remember it takes 1 bar of energy to go around a lap. You probably already spotted the error in the drive method that accepts laps. You can drive way more laps than our battery can handle. Let's fix it.
Add logic to the drive method so that it throws an IllegalArgumentException if there aren't enough bars to support the laps request. Remember it takes 1 bar of energy to go around a lap.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
if (barCount < lapsDriven){
throw new IllegalArgumentException("Too many laps");
}
}
}
3 Answers

Sean M
7,344 Pointsif the bar count is larger than then laps to complete, laps driven will be incremented, bar count will be decremented.
But if the amount of laps is larger than the battery/bar count, the program will throw a new illegal argument exception letting the driver know that there are not enough bars.
public void drive(int laps) {
if (laps <= barCount) {
lapsDriven += laps;
barCount -= laps;
} else {
throw new IllegalArgumentException("Not enough bars");
}
}
}

Lauren Moineau
9,483 PointsYou should have got the following error:
Bummer: Make sure you throw the exception before you change the barCount and lapsDriven fields.
We want to check that the battery has enough bar before we start driving, else there's no point in driving. So you need to move your if block to the top of the drive() method. Since we haven't started driving yet, we want to check the barCount against the laps count (not the lapsDriven):
public void drive(int laps) {
if (barCount < laps){
throw new IllegalArgumentException("Too many laps");
}
lapsDriven += laps;
barCount -= laps;
}
Hope this helps

Tashfin Shahid
1,298 Pointsthank you very much :)

Lauren Moineau
9,483 PointsYou're welcome :)
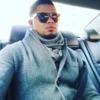
Yamil PƩrez
Courses Plus Student 2,054 Pointsnice and spice
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsYes, this is absolutely correct too. However, it is common (some may even say best) practice to use guard clauses to make the code cleaner and more readable. Basically, with guard clauses, you make those checks first, at the top of the method, so you can return or throw the necessary exception early, the rest of the code focusing on what the method is supposed to be doing. For example: