Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial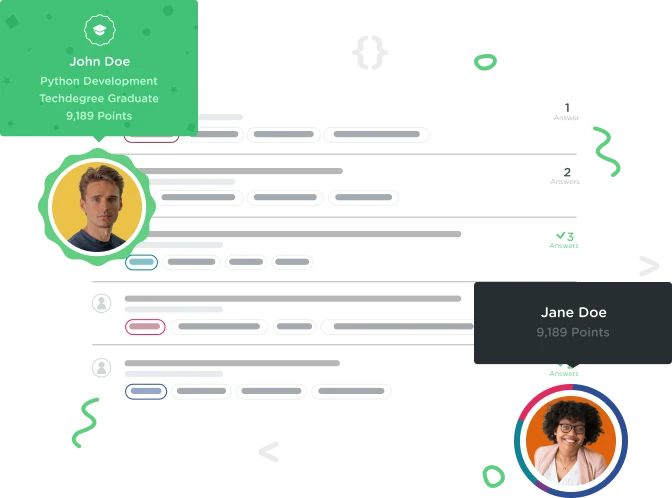
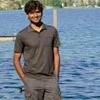
Lionel Victor
2,490 PointsException when caught print out the previous value of 'guess' and not the current
Below is my code
import random
def game():
#generate a random number
secret_num=random.randint(1 , 10)
#minimum number of guesses is set to a value
guesses = []
while len(guesses) < 5 :
try:
#guess the number from player
guess=int(input("Guess a number between 1 and 10:\n"))
except ValueError:
print("{} isn't a number !".format(guess))
except NameError:
print("{} isn't a number !".format(guess))
else:
#compare guess to secret number
if guess > secret_num:
print("too high")
guesses.append(guess)
elif guess < secret_num:
print("too low")
guesses.append(guess)
else:
print("number matched")
guesses.append(guess)
break
else:
print ("{} is your list of guesses".format(guesses))
print ("{} was the secret number".format(secret_num))
playagain = raw_input("Do you want to play again? Y/N:")
if playagain.lower() != "n" :
game()
else:
print("see you later")
game()
When executing the code: Lionels-Air:workspace Lionel$ python guess_number2.py Guess a number between 1 and 10: 23 too high Guess a number between 1 and 10: qwerty 23 isn't a number ! Guess a number between 1 and 10:
Question1: I was expecting it to return "qwerty isn't a number" rather it returned "23 isn't a number"
Question2:
Also if I try to enter a string in the very first try it give me the following "UnboundLocalError: local variable 'guess' referenced before assignment"
(execution shown below) Lionels-Air:workspace Lionel$ python guess_number2.py Guess a number between 1 and 10: qwerty Traceback (most recent call last): File "guess_number2.py", line 48, in <module> game() File "guess_number2.py", line 21, in game print("{} isn't a number !".format(guess)) UnboundLocalError: local variable 'guess' referenced before assignment
How do I avoid this ?
Thanks, -Lionel
1 Answer

Ryan S
27,276 PointsHi Lionel,
The problem might be that you are attempting to convert the input to an int in the same line. If you try to enter a string as your first guess, it will go to the ValueError block as expected but it will throw an error inside the block because it can't format the {} since guess isn't assigned to anything yet.
If you give it a number first, then try a string on another attempt, it will trigger the ValueError but guess will already have a value of the last number you tried. It won't be updated to the string you entered because it threw an error in the int conversion attempt.
To fix this, try separating the user input and the int conversion into two lines. For this you could use a separate variable, called user_input, for example, and define it outside of the try block. You can use the try block to check the int conversion.
while len(guesses) < 5 :
user_input = input("Guess a number between 1 and 10:\n")
try:
guess = int(user_input)
except ValueError:
print("{} isn't a number !".format(user_input))
except NameError:
print("{} isn't a number !".format(user_input))
Hope this helps.
Ismail KOÇ
1,748 PointsIsmail KOÇ
1,748 PointsThanks, i had same problem