Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial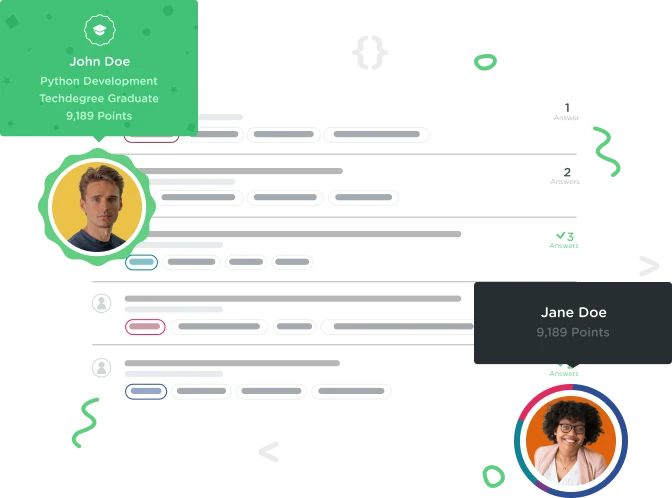

Enyang Mercy
Courses Plus Student 2,339 PointsExceptions challenge 1 throw exception on drive method
Throw an exception if there aren't enough bars to support lap request
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int newlaps = lapsDriven + barCount;
if (newlaps > MAX_BARS) {
throw new IllegalArgumentException("There aren't enough bars!!!!");
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi there
Basically all you have to do is to make sure that requested LAPS does not exceed the number of BARCOUNT. Thus we can state it in if statement as : if(laps > barCount) this is my proposed code: (if you want further explanation I add some below the code if you want more elaborate reason)
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("There aren't enough bars!!");
}
lapsDriven += laps;
barCount -= laps;
}
}
As mentioned in the challenge each lap will cost exactly one bar. Meaning the maximum lap than can be achieved is basically the number of bar that still available. The laps variable passed to the drive (int laps) method denotes how many laps the user specified. Thus to test whether it exceed the maximum number of laps that the battery can manage it should not exceed the number of bar available (this is denoted by barCount variable NOT the MAX_BAR constant). Remember the user cannot be guaranteed to get maximum battery level for instance if the Go Kart have been used before and have not been charge prior to the user turn.
Oh by the way it will be better if you run the if statement test above the other code since it will be more logical to do so in real life. If there still enough battery we will let the user to have a go. If it not then we need to warn them and stop them before they complaint that the battery is insufficient to get their desired laps.
I hope this will help you a little.