Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial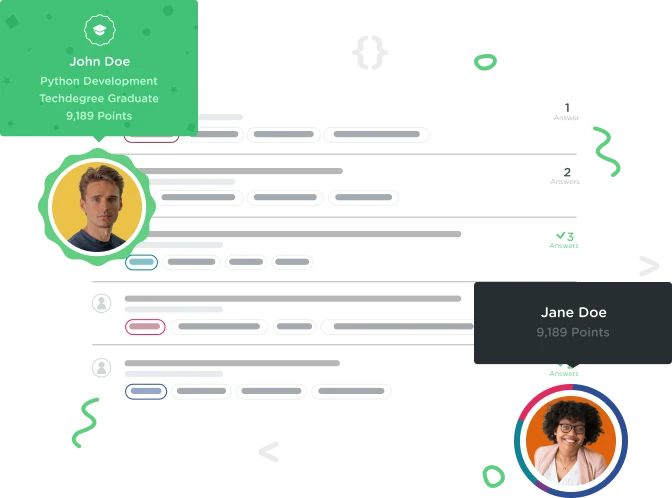

Ross Logan
8,131 PointsExceptions in Unit Testing (Best Practices)
In the Exceptions | Unit Testing in Java | tutorial video one of the comments that is made in the video is that each Test Case should throw the base exception rather than a specific exception (e.g. InvalidLocationException). Would someone please be able to explain why this is the case?
2 Answers
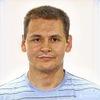
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsLooking for Unit Test Best Practices. Just found this awesome article
The section you are looking for is called "Do not write your own catch blocks that exist only to fail a test"
Quoting from there
Do not write your own catch blocks that exist only to fail a test
It is unnecessary to write your own catch blocks that exist only to fail a test because the JUnit framework takes care of the situation for you. For example, suppose you are writing unit tests for the following method:
final class Foo
{
int foo(int i) throws IOException;
}
Here we have a method that accepts an integer and returns an integer and throws an IOException if it encounters an error. Here is the wrong way to write a unit test that confirms that the method returns three when passed seven:
// Don't do this - it's not necessary to write the try/catch!
@Test
public void foo_seven()
{
try
{
assertEquals(3, new Foo().foo(7));
}
catch (final IOException e)
{
fail();
}
}
The method under test specifies that it can throw IOException, which is a checked exception. Therefore, the unit test won't compile unless you catch the exception or declare that the test method can propagate the exception. The second alternative is preferred because it results in shorter and more focused tests:
// Do this instead
@Test
public void foo_seven() throws Exception
{
assertEquals(3, new Foo().foo(7));
}
We declare that the test method throws Exception rather than throws IOException - see below for the reason. The JUnit framework will make sure that this test fails if any exception occurs during the invocation of the method under test - there's no need to write your own exception handling.
My addition
It is like you pointed best practice. If you know that you test method that potentially can throw an Exception, you better use throws Exception
signature. That is the best practice.
If you want to catch one use throws Exception
with expected
that's it :)

Ross Logan
8,131 PointsGood explanation and link. Thanks for this.