Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial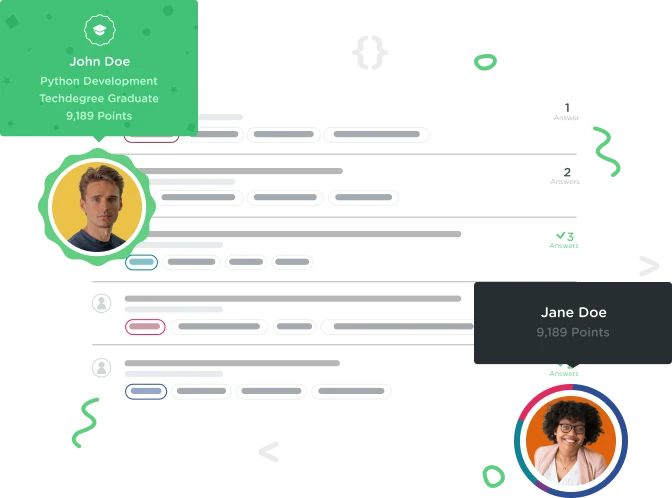

Jason Woo
11,730 PointsExcluding variables in search?
The address search results include the 'kind' variable. Which means if I search 'm', I'm going to get all contacts with 'Home' addresses without 'm's. How should I exclude this?
1 Answer
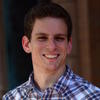
Jeff Lange
8,788 PointsIt isn't clear what you're asking. Can you clarify what you mean?
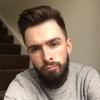
Matthew Wilkes
4,810 PointsHe wants to exclude the 'Kind' of address from the results, so if you were to search for an address that contained 'home' it would only contain the addresses that had it as part of their address i.e. 123 home lane. Currently it would show every 'home' address.
Jason Woo
11,730 PointsJason Woo
11,730 Points