Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial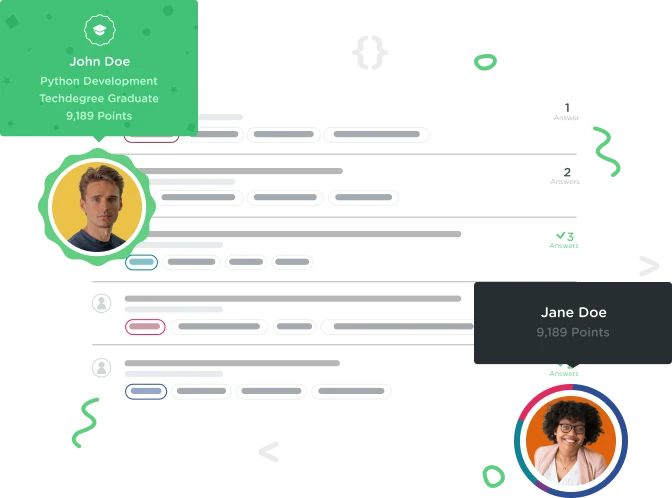

Justin Richie
Courses Plus Student 7,254 PointsExecuting Python code in 3.4.3 shell
From the python 3.4.3 shell, I am trying to use the Shopping Cart example from "Fun with FUNctions" and am having some trouble
shopping_list = []
def show_help(): print("What should we pick up at the store?") print("Enter DONE to stop. Enter SHOW to see current list")
def add_to_list(item): shopping_list.append() print("Added! List has {} items.".format(len(shopping_list)))
def show_list(): print("Here's your list:") for item in shopping_list: print(item)
show_help()
while True: new_item= input ("> ") if new_item == 'DONE':
break
add_to_list(new_item)
continue
show_list()
My ERROR is below:
================================ RESTART ================================
What should we pick up at the store? Enter DONE to stop. Enter SHOW to see current list Something Traceback (most recent call last): File "C:/Users/Justin/Documents/Python/function.py", line 24, in <module> add_to_list(new_item) File "C:/Users/Justin/Documents/Python/function.py", line 8, in add_to_list shopping_list.append() TypeError: append() takes exactly one argument (0 given)
any thoughts?
4 Answers

Gavin Ralston
28,770 PointsTake a look here at your function:
def add_to_list(item):
shopping_list.append()
print("Added! List has {} items.".format(len(shopping_list)))
Right now, you have the add_to_list function accepting an item for an argument. You want to add that item to your shopping list, so you'll need to pass it along to the append() function like so:
shopping_list.append(item)
Give that a try and see what happens.

Gavin Ralston
28,770 Pointsdef add_to_list(item):
shopping_list.append()
# etc, etc
The traceback tells you this:
shopping_list.append() TypeError: append() takes exactly one argument (0 given)
To get this message to go away, you need to provide an argument to append -- probably the item you passed in, like so:
shopping_list.append(item)
Hope that helps!
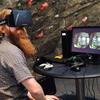
Jason Aylward
2,318 PointsI think you just need to tell shoppinglist what you would like to append:
shopping_list.append(item)

Justin Richie
Courses Plus Student 7,254 PointsSomething like this? I am new to python! THanks for the help.
shopping_list = []
def show_help(): print("What should we pick up at the store?") print("Enter DONE to stop. Enter SHOW to see current list")
def add_to_list(item): shopping_list.append() print("Added! List has {} items.".format(len(shopping_list)))
def show_list(): print("Here's your list:") for item in shopping_list: print(item)
show_help()
while True: new_item= input ("> ") if new_item == 'DONE':
break
add_to_list(new_item)
continue
show_list()
shopping_list.append(item)