Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial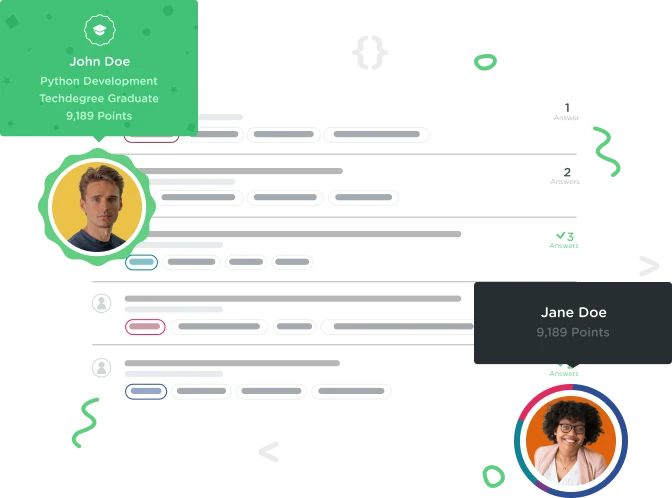

Graham Shunk
1,097 Pointsexiting
I have tried my code many different times, each one a little different, but no matter how many times I try my code, the same error message keeps popping up. It says: 'Didn't get an exit from start_movie', or something like that. Can someone please tell me what I am doing wrong!
import sys
var = input("Do you want to start the movie right now? ")
if var != "n" or "N":
print("Enjoy the show!")
else:
sys.exit()
4 Answers

Stefan Plรถtz
6,237 PointsIn addition to Alex's solution you can also do it like this
# Indicate that yes is the default value in case the user doesn't enter anything
var = input("Do you want to start the movie right now? (Y/n)")
# the lower() method will take the input string and convert it to lower case
if var.lower() != 'n':
print("Enjoy!")

Jing Zhang
Courses Plus Student 6,465 PointsThis should work:
import sys
var = input("Do you want to start the movie right now? ")
if var.lower() != "n":
print("Enjoy the show!")
else:
sys.exit()
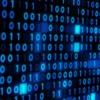
Alexander Davison
65,469 PointsYou can't use the "or" operator like this:
var != "n" or "N"
but you can use it like this:
var != "n" or var != "N"
So this means you should fix your condition, and then this should work:
import sys
var = input("Do you want to start the movie right now? ")
# I fixed the condition! :)
if var != "n" or var != "N":
print("Enjoy the show!")
else:
sys.exit()
Hope this helps and Happy coding! ~Alex

Graham Shunk
1,097 PointsI tried what you recommended, and I understand what I did wrong, but the same error message keeps popping up: Didn't get an exit from start_movie
. I still cannot understand what I did wrong. If it helps, here are the instructions for the code challenge:
Use input() to ask the user if they want to start the movie. If they answer anything other than "n" or "N", print "Enjoy the show!". Otherwise, call sys.exit(). You'll need to import the sys library.
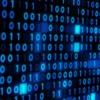
Alexander Davison
65,469 PointsHmmm... That's strange..
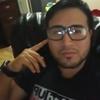
Frank Campos
4,175 PointsI had difficulties in this problem too. However, I fixed it, giving one enter to import sys and the rest of the code, too. I hope this help.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsGood one! I knew that you could do that, but I don't like to make my answers too complicated for new students