Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial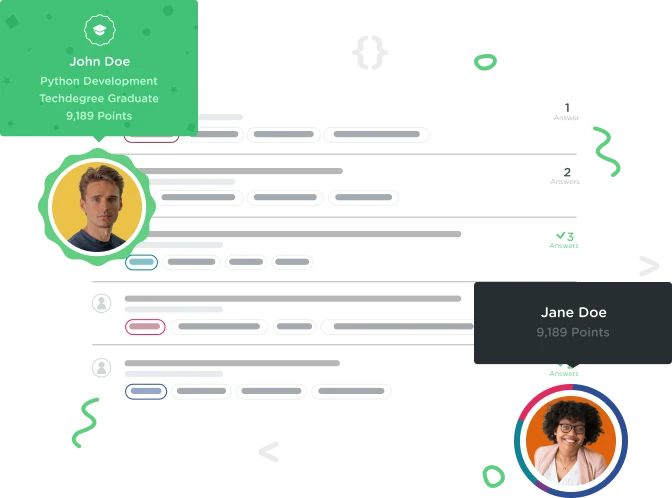
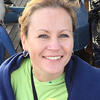
Jodi Engel
4,726 PointsExpanding the quiz - better solutions?
I tried to go a little further with this, changing the feedback based on how the user answered or if answers weren't provided. It took me forever to finally get it to work, so I'm just wondering if there was a different/better way of going about it. Here's what I came up with:
// 1. Create a multidimensional array to hold quiz questions and answers
let quiz = [
['Who is the POTUS?', 'Joe Biden'],
['What does "IPA" stand for?', 'Indian Pale Ale'],
['Which planet is closest to the sun?', 'Mercury'],
['Whose face was said to have launched 1,000 ships?', 'Helen of Troy']
];
// 2. Store the number of correct answers
let correctAnswers = 0;
// 2b. Store which questions were correct/incorrect
let correct = [];
let incorrect = [];
let notAnswered = 0;
/*
3. Use a loop to cycle through each question
- Present each question to the user
- Compare the user's response to answer in the array
- If the response matches the answer, the number of correctly
answered questions increments by 1
*/
for ( let i = 0; i < quiz.length; i++ ) {
let question = quiz[i][0];
let answer = quiz[i][1]
let response = prompt(question);
if (!response) {
notAnswered++;
} else if(response === answer) {
correctAnswers++;
correct.push(question);
} else {
incorrect.push(question);
}
}
function createListItems(arr) {
let items = '';
for ( let i = 0; i < arr.length; i++ ){
items += `<li>${arr[i]}</li>`;
}
return items;
}
console.log(correct.length);
console.log(incorrect.length);
console.log(quiz.length);
// 4. Display feedback based on the number of correct answers
if (notAnswered === 0 && correct.length === quiz.length) {
feedback = `<h1>Awesome! You got everything right!</h1>`
} else if (notAnswered === 0 && incorrect.length === quiz.length) {
feedback = `<h2>Sorry. You got all the questions wrong.</h2`
} else if (notAnswered === 0 && correct.length > 0 && correct.length < quiz.length) {
feedback = `
<h1>You got ${correctAnswers} question(s) correct.</h1>
<h2>These are the questions you answered right:</h2>
<ol>${createListItems(correct)}</ol>
<h2>These are the questions you answered wrong:</h2>
<ol>${createListItems(incorrect)}</ol>
`;
} else {
feedback = `<h2>Sorry. You must provide and answer to all the questions.</h2>`
}
document.querySelector('main').innerHTML = feedback;
2 Answers

Blake Larson
13,014 PointsLooks really good! I added a few comments if you're interested in what could be added or removed
let quiz = [
['Who is the POTUS?', 'Joe Biden'],
['What does "IPA" stand for?', 'Indian Pale Ale'],
['Which planet is closest to the sun?', 'Mercury'],
['Whose face was said to have launched 1,000 ships?', 'Helen of Troy']
];
// ***Removed correct variable and just use correct.length****
// 2b. Store which questions were correct/incorrect
let correct = [];
let incorrect = [];
let notAnswered = 0;
for ( let i = 0; i < quiz.length; i++ ) {
let question = quiz[i][0];
// **** since they are all strings you can make the answer all lowercase for
// edge cases when comparing ****
let answer = quiz[i][1].toLowerCase();
let response = prompt(question);
if (!response) {
notAnswered++;
} else if(response.toLowerCase() === answer) {
correct.push(question);
} else {
incorrect.push(question);
}
}
function createListItems(arr) {
let items = '';
for ( let i = 0; i < arr.length; i++ ){
items += `<li>${arr[i]}</li>`;
}
return items;
}
// *** Check if any went unanswered first so you don't have to repeat
// the `notAnswered === 0` condition ****
if(notAnswered > 0) {
feedback = `<h2>Sorry. You must provide and answer to all the questions.</h2>`
} else {
if (correct.length === quiz.length) {
feedback = `<h1>Awesome! You got everything right!</h1>`
} else if (incorrect.length === quiz.length) {
feedback = `<h2>Sorry. You got all the questions wrong.</h2>`
} else {
// **** If all are answered and you didn't get 100% 0R 0% correct ****
// **** ${correct.length} instead of correct ****
feedback = `
<h1>You got ${correct.length} question(s) correct.</h1>
<h2>These are the questions you answered right:</h2>
<ol>${createListItems(correct)}</ol>
<h2>These are the questions you answered wrong:</h2>
<ol>${createListItems(incorrect)}</ol>
`;
}
}
document.querySelector('main').innerHTML = feedback;
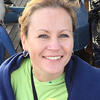
Jodi Engel
4,726 PointsAhhh - thank you so much! Definitely a little cleaner - which is what I'm trying to work on for sure! Thanks again!