Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial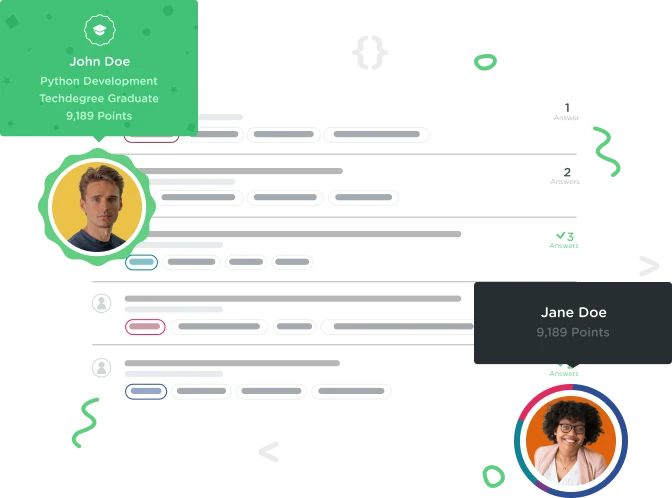

Simone Watson
2,072 PointsExpected "Player 1: 5; Player 2: 2". Got "Player 1: 5, Player 2: 10".
Please help me figure out the tuple aspect of this question. I'm not sure how to define self.score, and I think that's why my code is messing up...
2 Answers
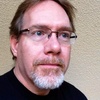
Chris Freeman
Treehouse Moderator 68,454 PointsTwo issues:
from game import Game
class GameScore(Game):
pass
def __str__(self):
# self.score = (5,10) # <-- comment this line. not needed
self.player_1, self.player_2 = self.score
return "Player 1: {}; Player 2: {}".format(self.player_1, self.player_2) # <-- replace comma with semi-colon
The grader can be so picky!

Simone Watson
2,072 PointsHere's my code...
from game import Game
class GameScore(Game):
pass
def __str__(self):
self.score = (5,10)
self.player_1, self.player_2 = self.score
return "Player 1: {}, Player 2: {}".format(self.player_1, self.player_2)
[MOD: fixed code formatting with backtick ` instead of single quote ` -cf]
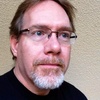
Chris Freeman
Treehouse Moderator 68,454 PointsI noticed you have a comma (,) instead of a semi-colon (;) in the return
string.
Also, you do not want to set the value of self.score
. It will be inherited from the Game
class.
Niels Dhollander-Barclay
4,674 PointsNiels Dhollander-Barclay
4,674 PointsI found this solution after I was trying to pass the following return statement:
return "Player 1: {}; Player 2: {}".format(self.score)
Why can't I just pass the self.score tuple in my return statement?
Edit: Thank you for your solution, I like the last one referencing the indices the best! So much elegant!
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsThe error you maybe seeing is due to a mismatch between the number of fields
{}
and the number of arguments provided.Try either:
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsI found two other ways to use the
format
statement: pass an object so you can reference attributes directly:Even more dense is to reference off of positional arguments:
self.score
is in positional position 0, the references0[0]
and0[1]
reference the first and second item of the object.