Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial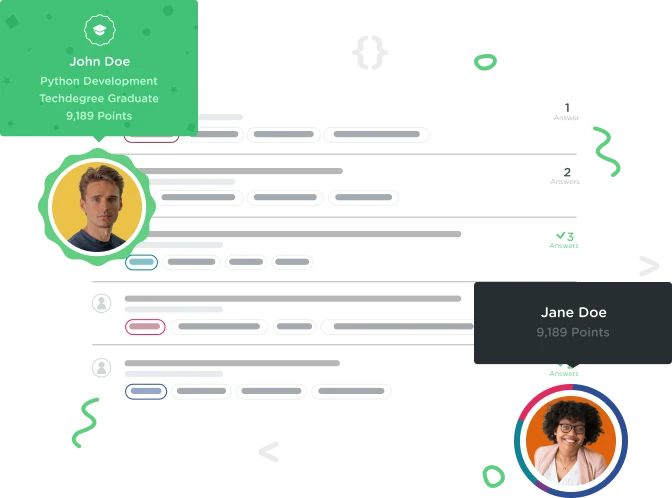

Kevin Ohlsson
4,559 Pointsexperiement with app help
I dont know how to make template literal not pring as string
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body id="bodyPage">
<h1 id="heads"> js hello</h1>
<input type="what" id="myTextInput">
<button id="changeColorButton">change headline color</button>
<input type="text" id="myStyleInput">
<button id="changeStyleButton">Change Style</button>
<button id="resetColorButton">Default color</button>
<script src="app.js"></script>
</body>
</html>
js
const myHeading = document.getElementById("heads");
const changeColorButt = document.getElementById("changeColorButton");
const textInput = document.getElementById("myTextInput");
const resetColorButton = document.getElementById("resetColorButton");
let styleInput = document.getElementById("myStyleInput");
changeColorButt.addEventListener("click", () => {
myHeading.style.color = textInput.value;
});
resetColorButton.addEventListener("click", () => {
myHeading.style.color = "black";
});
changeStyleButton.addEventListener("click", () => {
return `myHeading.style.${styleInput.value}`
});
input in changeStyleButton
direction = "rtl"
in console:
`myHeading.style.${styleInput.value}`
3 Answers

Andrew Hinkson
Full Stack JavaScript Techdegree Graduate 25,822 PointsYea sorry my bad wasn't paying attention. So yea from what I am seeing you can't dynamically change dot notation with literals. One way I found that you can make your idea work is this:
Adding a button to your html
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body id="bodyPage">
<h1 id="heads"> js hello</h1>
<input type="what" id="myTextInput">
<button id="changeColorButton">change headline color</button>
<input type="text" id="myStyleInput">
<input type="text" id="myStyleValue"> // this one right here
<button id="changeStyleButton">Change Style</button>
<button id="resetColorButton">Default color</button>
<script src="app.js"></script>
</body>
</html>
Then adding this to your js would make it to where you could dynamically change your code to anything your wanting i would imagine within reason :)
changeStyleButton.addEventListener("click", () => {
var style = document.getElementById("myStyleInput").value;
var value = document.getElementById("myStyleValue").value;
myHeading.style[thing] = style;
});
In the first box type what you want to do like direction or whatever then the value you want to change it too in the second.
Im still learning all this so I am hoping that this is helping.

Joel Mora
9,859 PointsAndrew Hinkson hit it right on the dot I believe. I also decided to try and create a flexible style changing input field and came up with almost the exact same solution as Mr. Hinkson.
I am posting my code below just because I already have it so, why not? Maybe it might help to have 2 perspectives.
HTML:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<!--This Text field will allow you to select type of style (ex. direction, color, backgroundColor, ect..-->
<label for="myStyleSelection">Select which style you would like to change on the header:</label>
<input type="text" id="myStyleSelection"><br>
<!--This Text field will allow you to select a value for the style you previously selected (ex. 'rtl' (if you entered direction in the previous textField-->
<label for="myStyleValue\">Select what value you would like to change your style to instead:</label>
<input type="text" id="myStyleValue">
<button id="myButton">Change style of Heading</button>
<script src="app.js"></script>
</body>
</html>
Javascript:
const myHeading = document.getElementById('myHeading');
const myButton = document.getElementById('myButton');
const myStyleSelection = document.getElementById('myStyleSelection');
const myStyleValue = document.getElementById('myStyleValue');
myButton.addEventListener('click', () =>{
myHeading.style[myStyleSelection.value] = myStyleValue.value;
})

Andrew Hinkson
Full Stack JavaScript Techdegree Graduate 25,822 PointsI dont believe you can actually use a literal with dot notation. I could be wrong but the only way I could get your code to work was to do this:
changeStyleButton.addEventListener("click", () => {
var style = document.getElementById("myStyleInput").value;
myHeading.style.direction = style;
});
I know it doesnt answer the question but hopefully this helps.

Kevin Ohlsson
4,559 Pointsthank you Andrew!
Your code changes the direction property, right?
If i wanted to change whatever comes after style, is that possible? Say i wanted to instead of "direction" change "textAlign" property in the same field.
myHeading.style.${changeToAnyStyleProperty}
Below snippet did not work. I dont understand why it works with the color property. Is style not a property too?
changeStyleButton.addEventListener("click", () => {
myHeading.style = styleInput.value
});
maybe not possible to do this dynamically without functions