Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial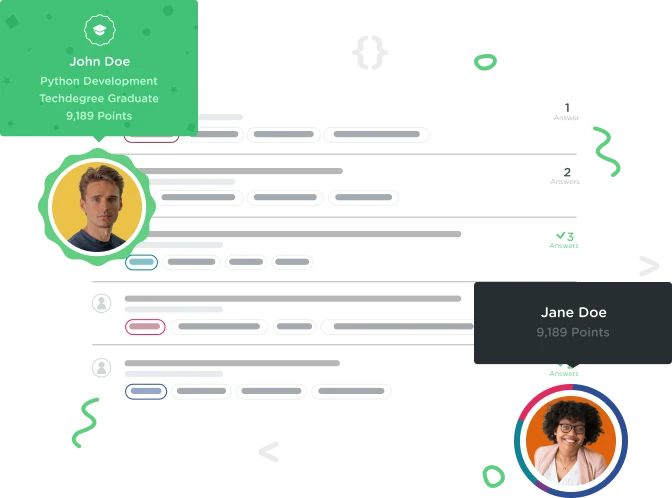
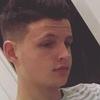
Nick Evershed
570 PointsExplain code?
for letter in "You got this!": if letter in "oh": print(letter)
5 Answers

Jennifer Membreno
1,705 PointsI hope this explanation helps.
for letter in "You got this!":
this first line iterates through each value in the string and assigns it the letter variable
if letter in "oh":
the second line is an if statement, which checks to see if each iterated value that is stored in the letter variable is in (or matches any of the values in) the string "oh"
print(letter)
if the iterated value matches either "o" or "h", then it is "o" or "h" is printed out depending on which value was matched.
if "o" matches "o", "o" is printed out. If "h" matches "h", "h" is printed out. If nothing matches "o" or "h", nothing is printed out.
"Y" does not equal "o" or "h", so nothing gets printed out. Now we move to the next value.
"o" is, in fact, equal to "o", so that DOES get printed out.
This continues until each value of the string "You got this!" has been evaluated.
Therefore, the final output after the loop has finished is:
o
o
h
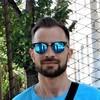
Oszkár Fehér
Treehouse Project ReviewerHi Nick. Looping trough a string it's like looping trough a list
string = 'Yes you got this' == ['Y', 'e', 's', ' ' , 'y', 'o', 'u', ' ', 'g', 'o', 't', ' ', 't', 'h', 'i', 's', '!']
With for loop:
for each_letter in string:
if each_letter in 'oh': ---> Where 'oh' it's a list of characters, ['o', 'h'], it checks if each_letter is in the list
print(each_letter)
so the result will be o - from "you", o - from "got" and h - from "this", where the output will be
o
o
h
But be careful with this, string can be a list but list it's not a string! I hope this helps you to understand better. Happy coding.
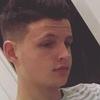
Nick Evershed
570 PointsI won't lie this confused me more, what even is oh?
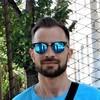
Oszkár Fehér
Treehouse Project ReviewerThe oh
it's a collection of string which in this case it's ['o', 'h']
for each_letter in 'oh':
can be as
for each_letter in ['o', 'h']:
and the each_letter
is one letter from the string
string or list.
With the for
loop we iterate the string
variable which is also a list
If you still need further explination please let me know and I will explain more detailed the for
loop and why the string
acting like a list.
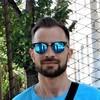
Oszkár Fehér
Treehouse Project Reviewer"oh" it's just a list of characters or string of characters what the quiz gives to check out if the sentence contains the letter "o" or "h". I suggest you do the Python Basics 2015 version as well. You can run that little code what i wrote last time(in your python editor or python shell on your computer), without the comment what i wrote after the arrow, and try to change the "oh" with something else, "os" or "ti" or even more letters and you will see the outcome. This part is one of the easiest part of basics. If it's still unclear, please don't hesitate to write. Good learning.

Siddharth Pande
9,046 Pointsfor letter in "You got this!": if letter in "oh": print(letter) when this code runs it will take each letter from the string "You got this!", one by one and check if that letter belongs to another string "oh" and if that letter is present/belongs to the string "oh" then print that letter on the screen. This code may also be written as: for character in "You got this!": if character in "oh": print(character) and character will include all the letters, spaces, special characters from the string "You got this!". There are two strings used in this program "You got this!" and "oh". and letter or character is a variable that can store any string or number in it as and when required by the program. String is a type of data. There are different types of data that are used in programming. Don't worry just keep on programming and you will understand every bit day-by-day. We have all gone through the phase you are going through. If you have any more questions i am happy to help. You may contact me via siddharthpande@me.com if needed.
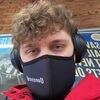
Szymon Dabrowski
2,207 PointsHow I explained this to myself is like this:
>>> for letter in "You got this!":
... if letter in "oh":
... print(letter)
...
o
o
h
Basically the "you got this!" is just a list of characters let's say. and the "oh" is looking at the list from left to right and it wants to see how many times it can find those letters. Then print them out one by one. so with "oh" as you go along "You got this". You have an O in "you" then an O in "got" and an H in "this!".
So to reinforce my theory I changed "oh" to something else. I changed it to "gt". so from left to right, it will search for those letters then print them out one by one. So in "got" there was a G as well as a T. Then in "this!" there was a T so it printed g t t
>>> for letter in "You got this!":
... if letter in "gt":
... print(letter)
...
g
t
t
I hope this helps
Allison Schoonover-Wells
1,151 PointsAllison Schoonover-Wells
1,151 PointsThis was so helpful! Thank you!