Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial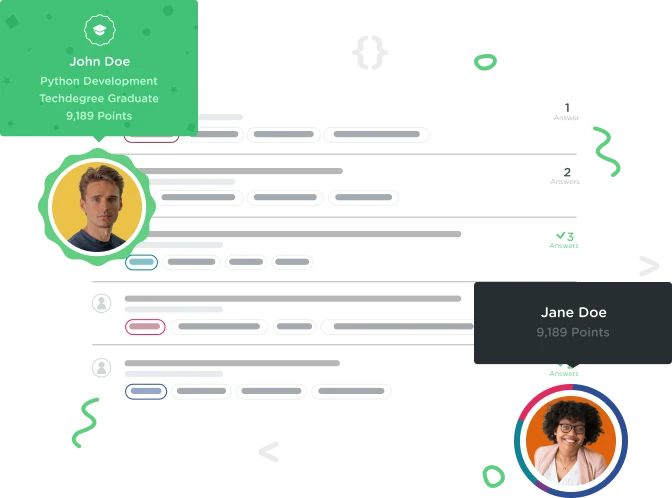
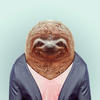
zlatkap
21,494 PointsExplain Concept of creating entity of Class with .class - in this case Category.class
I still do not get how to use it and where what what is the concept or what does this do specifically. in this case (List<Category> categories = session.createCriteria(Category.class).list();) .... I saw it in so many videos and tutorials and still not getting it - what is the specific role of Category.class and what does it represents?
1 Answer
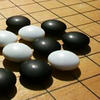
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsWell, it's about the Criteria API and they could probably make five different courses on the subject and they still wouldn't have covered it.
But here's the short version: The Criteria API is one of the components of the Java Persistence API (JPA) which defines how a Java app and a database should work together. Java only knows about objects, while the database has tables with rows that can be queried with SQL. Criteria is one of those things that build bridges between the two. With Criteria, you can build a query using Java objects, and it will transform it into a valid SQL query in the background. Then it will take the result of the SQL query (a bunch of rows from a database table) and turn them back into Java objects that you can use in your code.
The line that you quote basically just sends a query to the database to get all the data from the category table, then turn them into Category objects and put them into a Java List. You pass it the Category.class as a parameter to say that you want Java objects of the type Category. Then Hibernate does the rest of the job: from the configuration of your app, it knows how Category objects are mapped to the database.
You might also encounter this updated version of the code, which looks more complicated but does exactly the same, only with the latest version of Hibernate and JPA:
public List<Gif> findAll() {
Session session = sessionFactory.openSession();
// Create CriteriaBuilder
CriteriaBuilder builder = session.getCriteriaBuilder();
// Create CriteriaQuery
//Here you create a new query. The "Gif.class" means you want the query to return Gif objects
CriteriaQuery<Gif> criteria = builder.createQuery(Gif.class);
//Specify the root of the query, the entity that's the basis for the query.
//Here you just want all Gifs from the gif table, so it's obvious, but it can be more complicated with complex queries
criteria.from(Gif.class);
// Execute the query, then put all the data in a Java List
List<Gif> gifs = session.createQuery(criteria).getResultList();
session.close();
return gifs;
}
Honestly, I tried to understand how Criteria really works beyond the super simple case above, and it's pretty overwhelming. You won't encounter Criteria in Treehouse courses beyond what I just told you about, though. There are other ways to query data from the database. One of them is so called "Typed Queries" with JPQL. It has its drawbacks but is definitely easier. And in your next Spring course I believe you'll meet Spring Data JPA, which makes querying data so easy you almost forget there's a database somewhere in the background.