Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial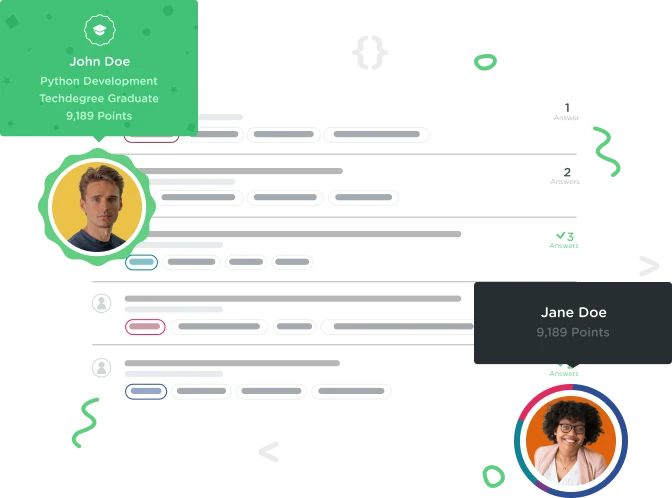

William J. Terrell
17,403 PointsExploring the Java Collection Framework - Array Lists Challenge
The question is as follows:
"Let's add a method that returns a list of all links in the body of the document. All words that start with http should be considered links. Name it getExternalLinks.
NOTE: Remember to import the needed classes from java.util. HINT: We already made the getWords method, we should use it!"
I feel like I should be close, but I get the following when trying to run the code below:
./com/example/BlogPost.java:38: error: cannot find symbol
return getWordsPrefixedWith("http");
^
symbol: method getWordsPrefixedWith(String)
location: class BlogPost
./com/example/BlogPost.java:45: error: cannot find symbol
if (word.startsWith(prefix)) {
^
symbol: variable word
location: class BlogPost
./com/example/BlogPost.java:46: error: cannot find symbol
results.add(word);
^
symbol: variable word
location: class BlogPost
Note: JavaTester.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
3 errors
On top of that, I'm still not entirely sure how this all works... (Am I making it more complicated than it really is?)
Any assistance would be greatly appreciated. I've left my "steps" in comments in the code below.
Thanks!
package com.example;
import java.io.Serializable;
import java.util.ArrayList; // Added this...
import java.util.Arrays; // ...and this...
import java.util.Date;
import java.util.List; //..and this...
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public List<String> getWords() { // Changed this from String[] to List<String>...
String[] words = mBody.split("\\s+"); //Added this using the code that was on the next line...
return Arrays.asList(words); // And added this...
}
// Added this...
public List<String> getLinks() {
return getWordsPrefixedWith("http");
}
// Then here's the rest...
public List<String> getExternalLinks(String prefix) {
List<String> results = new ArrayList<String>();
for (String words : getWords()) {
if (word.startsWith(prefix)) {
results.add(word);
}
}
return results;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
2 Answers

William J. Terrell
17,403 PointsAha! This got it! :)
package com.example;
import java.io.Serializable;
import java.util.ArrayList; // Added this...
import java.util.Arrays; // This...
import java.util.Date;
import java.util.List; // And this...
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
// Changed this, using the example from the video...
public List<String> getWords() {
String[] words = mBody.split("\\s+");
return Arrays.asList(words);
}
// Added this...
public List<String> getExternalLinks() {
return getWordsPrefixedWith("http");
}
// And here's the rest...
public List<String> getWordsPrefixedWith(String prefix) {
List<String> results = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith(prefix)) {
results.add(word);
}
}
return results;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
Though I still don't feel like I fully understand how it works... I kind of feel like I'm staring into an engine, and I can see that this part turns that part but...it's like I'm just zomb-ing out in front of the machine... :\
By the way, is it considered gauche for one to select his or her own answer as the "Best Answer"?

Nirbhay Agarwal
2,852 Pointspackage com.example;
import java.io.Serializable; import java.util.Date; import java.util.Arrays; import java.util.ArrayList; import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable { private String mAuthor; private String mTitle; private String mBody; private String mCategory; private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) { mAuthor = author; mTitle = title; mBody = body; mCategory = category; mCreationDate = creationDate; }
public int compareTo(BlogPost other) { if (equals(other)) { return 0; } return mCreationDate.compareTo(other.mCreationDate); }
public List<String> getWords() { String[] words = mBody.split("\s+"); return Arrays.asList(words); } public List<String> getExternalLinks() { List<String> docBody = new ArrayList <String>(); for(String words : getWords()) { if(words.startsWith("http")) { docBody.add(words); } } return docBody; }
public String getAuthor() { return mAuthor; }
public String getTitle() { return mTitle; }
public String getBody() { return mBody; }
public String getCategory() { return mCategory; }
public Date getCreationDate() { return mCreationDate; } }
Cory Carlson
5,275 PointsCory Carlson
5,275 Pointsimport java.util.List; // And this...
Thanks, I didnt have that part imported and was getting the same error.