Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial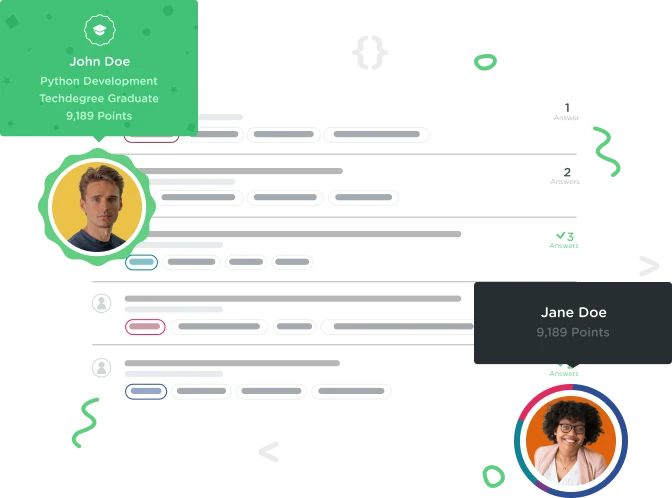

William J. Terrell
17,403 PointsExploring the Java Collection Framework - Sets Challenge
I have a feeling that I'm close (please correct me if I am wrong), but I don't know what to put for the values that, in the video, Craig Dennis put for:
for (Treet treet : treets)
What are these values, and how do they work in the "formula" of the way the code is set up?
My code for the challenge is below; I added comments where the values in question are supposed to go (where it says "Something").
Any assistance and further explanation of how this all works would be greatly appreciated. :)
Thanks!
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.HashSet; // Added this...
import java.util.Set; //...this...
import java.util.TreeSet; //...and this...
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
//Then the rest is here
Set<String> getAllAuthors = new TreeSet<String>();
for (BlogPost /* Something */ : /*Something */) {
getAllAuthors.addAll(/* Something */.getAuthor());
}
}
1 Answer
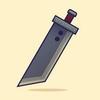
Allan Clark
10,810 Points//Then the rest is here
Set<String> getAllAuthors = new TreeSet<String>();
for (BlogPost /* Something */ : /*Something */) {
getAllAuthors.addAll(/* Something */.getAuthor());
}
First we are missing the method declaration here. It should be public and return a Set of Strings. Then we have a for-each loop. The first "something" is just a variable name for the specific BlogPost we will be using on each cycle of the loop. The second "something" is the collection that the for each loop is looping through. So far we should have something like this:
public Set<String> getAllAuthors() {
Set<String> allAuthors = new TreeSet<String>();
for(BlogPost post : mPosts){
// code to get the author and add it to the Set
}
return allAuthors;
}
Now we just need the code for inside the for-each loop. We are using the variable 'post' as the container for the element selected in each loop cycle. So we should just need to get the author of 'post' and add it to the Set. Of course returning the set at the end.
for(BlogPost post : mPosts){
allAuthors.add(post.getAuthor());
}
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsThanks! This code got it:
So, if I understand correctly, for one, I hadn't defined it (maybe I thought I had defined it on the line where I made the "new" one, where I had "Set<String> getAllAuthors = new TreeSet..." in the original code?), for two, I wasn't returning anything (should have caught that), and for three, that "post" (in the for loop) is a variable/key/something that we define right there, in the method itself? (So for that "something", it doesn't necessarily matter what you call it? )
Thanks!
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsOr, on that for-loop, is it:
Something along those lines? :)
Thanks!