Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial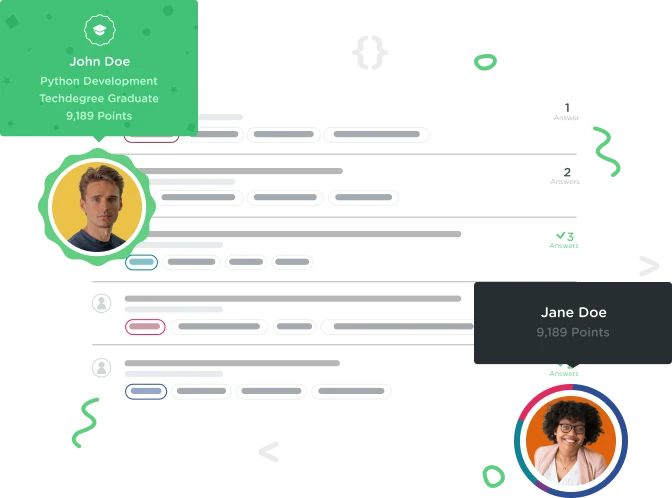
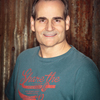
Bill Dowd
7,681 PointsExtending a class, but a fatal error, the parent is not found
I'm extending GenericObject.phpm to another class, payees.phpm, but I am getting a fatal error, "Class 'GenericObject' not found." I've used this method for years, but something has changed and is now a problem. I'll include the code for the top of each class file. I would appreciate someone setting me straight on this.
Thanks, Bill
<?php
include_once('sql.phpm');
class GenericObject {
# Member Variables
private $id;
private $insert_id;
<?php
class Payee extends GenericObject {
private $result_row;
private $id;
( ! ) Fatal error: Class 'GenericObject' not found in C:\wamp\www\AblePay_private\payee.phpm on line 2 Call Stack
Time Memory Function Location
1 0.0016 278904 {main}( ) ..\payees.php:0 2 0.0037 358880 include_once( 'C:\wamp\www\AblePay_private\payee.phpm' ) ..\payees.php:4
2 Answers
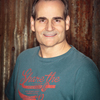
Bill Dowd
7,681 PointsIn order for a class to be extended, because it just doesn't magically happen, either include_once('parent_class'); in the extended class, which is the best way, or be sure you include_once('parent_class'); before you include_once('child_class'); in the file where you need to use the class files.
<?php
require_once('GenericObject.phpm');
class Payee extends GenericObject {
// ...
}
?>

Chris Adamson
132,143 PointsIt looks like you need to close out your class definitions with a bracket, }
<?php
include_once('sql.phpm');
class GenericObject {
# Member Variables
private $id;
private $insert_id;
}
class Payee extends GenericObject {
private $result_row;
private $id;
}
?>
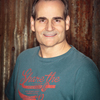
Bill Dowd
7,681 PointsThank you Chris. I didn't show it, but they both are. Here is the complete code for GenericObject:
<?php
include_once('sql.phpm');
class GenericObject {
# Member Variables
private $id;
private $insert_id;
private $table_name;
private $database_fields;
private $loaded;
private $modified_fields;
private $stmt;
private $insertstmt;
# Methods
public function Reload() {
$sql = new sql(0);
$id = $this->id;
$table_name = $this->table_name;
$sql->Query("SELECT * FROM $table_name WHERE id = $id");
$result_fields = $sql->GetRowHash(0);
$this->database_fields = $result_fields;
$this->loaded = 1;
if (sizeof($this->modified_fields) > 0) {
foreach ($this->modified_fields as $key => $value) {
$this->modified_fields[$key] = false;
}
}
}
private function Load() {
$this->Reload();
$this->loaded = 1;
}
public function ForceLoaded() {
$this->loaded = 1;
}
public function GetField($field) {
if ($this->loaded == 0) {
$this->Load();
}
return $this->database_fields[$field];
}
public function GetAllFields() {
if ($this->loaded == 0) {
$this->Load();
}
return($this->database_fields);
}
public function GetID() {
return $this->id;
}
public function GetInsertID() {
return $this->insert_id;
}
public function GetStmt() {
return $this->stmt;
}
public function GetInsertStmt() {
return $this->insertstmt;
}
public function Initialize($table_name, $tuple_id = "") {
$this->table_name = $table_name;
$this->id = $tuple_id;
}
public function SetField($field, $value) {
if ($this->loaded == 0) {
if ($this->id) {
$this->Load();
}
}
$this->database_fields[$field] = $value;
$this->modified = 1;
$this->modified_fields[$field] = true;
}
public function Destroy() {
$id = $this->id;
$table_name = $this->table_name;
if ($id) {
$sql = new sql(0);
$stmt = "DELETE FROM $table_name WHERE id = $id";
$sql->query($stmt);
}
}
public function Save() {
$id = $this->id;
$table_name = $this->table_name;
$sql = new sql(0);
if (!$id) {
$this->loaded = 0;
}
if ($this->loaded == 0) {
# assume this is a new entity
$stmt = "INSERT INTO " . $table_name ." (";
foreach ($this->database_fields as $key => $value) {
if (!is_numeric($key)) {
$key = str_replace("'", "\'", $key);
if ($value != "") {
$stmt .= $key . ", ";
}
}
}
# Chop last comma
$stmt = substr($stmt,0,strlen($stmt)-2);
$stmt .= ") VALUES (";
foreach ($this->database_fields as $key => $value) {
if (!is_numeric($key)) {
if ($value != "") {
$value = str_replace("'", "\'", $value);
$stmt .= "'$value', ";
}
}
}
# Chop last comma
$stmt = substr($stmt,0,strlen($stmt)-2);
$stmt .= ")";
//print "<p>" . $stmt . "</p>";
//exit();
}
else {
$stmt = "UPDATE " . $table_name ." SET ";
foreach ($this->database_fields as $key => $value) {
if (!is_numeric($key)) {
if ($this->modified_fields[$key] == true) {
// $value = str_replace("'", "\'", $value);
if ($value == "") {
$stmt .= "$key = NULL, ";
}
else {
$stmt .= "$key = '$value', ";
}
}
}
}
# Chop last comma and space
$stmt = substr($stmt,0,strlen($stmt)-2);
$stmt .= " WHERE id = $id";
}
$return_code = $sql->Query($stmt, 1);
$this->stmt = $stmt;
if ($this->loaded == 0) {
# Try to get the ID of the new tuple.
// $stmt = "SELECT MAX(id) AS id FROM $table_name WHERE ";
$stmt = "SELECT id FROM $table_name WHERE ";
foreach ($this->database_fields as $key => $value) {
if (!is_numeric($key)) {
if ($value) {
if ($this->modified_fields[$key] == true) {
$value = str_replace("'", "\'", $value);
$stmt .= "$key = '$value' AND ";
}
}
}
}
# Chop last " AND " (superfluous)
$stmt = substr($stmt,0,strlen($stmt)-5);
$this->insertstmt = $stmt;
//print "<p>" . $stmt . "</p>";
//exit();
$sql->Query($stmt);
$result_rows = $sql->GetRowHash(0);
$proposed_id = $result_rows['id'];
if ($proposed_id > 0) {
$this->loaded = 1;
$this->insert_id = $proposed_id;
return true;
}
else {
return false;
}
}
return($return_code);
}
}
?>
Bill Dowd
7,681 PointsBill Dowd
7,681 PointsHere is some more information: I created empty class files with only the class name and the { } and another to extend, nothing is working. No class file is seeing another class file. I'm running my development environment in WAMP. Are there settings in PHP or Apache that I need to address to get class files working?