Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial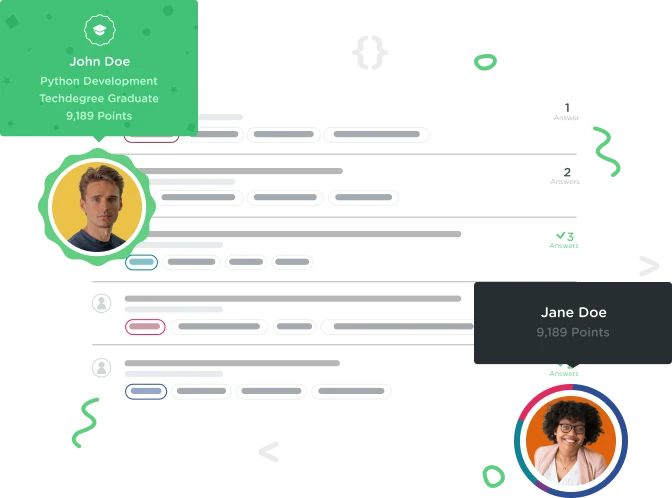

Chris Craigman
13,659 PointsExtending off parent class
https://teamtreehouse.com/library/extending-objectoriented-php/inheritance/extend-a-class
Extending from a parent class allows the child to inherit all attributes and abilities of the parent. Step 1: Add a new method to the Developer class named displayUserSkills. Step 2: Use the 'name' from the PARENT class, and the 'skills' from the CURRENT class, to replace the <PLACEHOLDERS> and RETURN following string (NOTE that skills separated with a comma and space): <NAME> has the following skills: <SKILL1>, <SKILL2>, <SKILL3> Example Output: Alena has the following skills: PHP, MySQL, HTML
I'm not sure what I'm doing wrong, all it tells me when I try submitting my answer is try again and not a hint towards what I'm doing wrong, hopefully one of you guys will be able to help me out.
Thank you in advance.
<?php
//do not modify this file
class User
{
protected $name;
protected $title;
public function __construct($name = null, $title = null) {
$this->name = $name;
$this->title = $title;
}
public function __toString() {
return $this->getSalutation();
}
public function getSalutation() {
return $this->title . " " . $this->name;
}
public function getName() {
return $this->name;
}
public function setName($name) {
$this->name = $name;
}
public function getTitle() {
return $this->title;
}
public function setTitle($title) {
$this->title = $title;
}
}
<?php
//add new child class here
class Developer extends User
{
protected $skills = array();
public function getSkills()
{
return $this->skills;
}
public function setSkills($skills)
{
$this->skills = $skills;
}
public function displayUserSkills()
{
return $this->getSkills();
}
$Developer1 = new Developer;
protected $skills = array("PHP","MySQL","HTML");
$Developer1 -> getSkills($skills);
$Developer1 -> setName("Alena");
echo $Developer1->getName . " has the following skills " . implode($Developer1->displayUserSkills());
}
Ray Knag
8,110 PointsRay Knag
8,110 PointsI am using this, and getting incorrect output, I feel like I am not looping through the array to add commas as the formatting dictates, but I am at a bit of a loss about what to do from here. Might be I just need to take a step back and come back later but if anyone has any suggestions they would be much appreciated.
I was able to track down the correct code in another post, the solution that I found is detailed below. It turns out the test does not want what is displayed in the example, it just wants you to set it up so that it will output something similar with no example. I removed the
protected $name = "Alena";
and"PHP, ","MySQL, ","HTML"
inside of the array. The thing that was throwing me off was theparent::getName()
as it was not covered in the lesson and I am pretty sure the individual submitting the response had a little more knowledge.After a little more playing with the code, I was able to find that this also works, without the
parent::getName()
line. You just have to remember to setprotected $name;
for the child class as it will use the info from the parent class like in the video examples.