Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial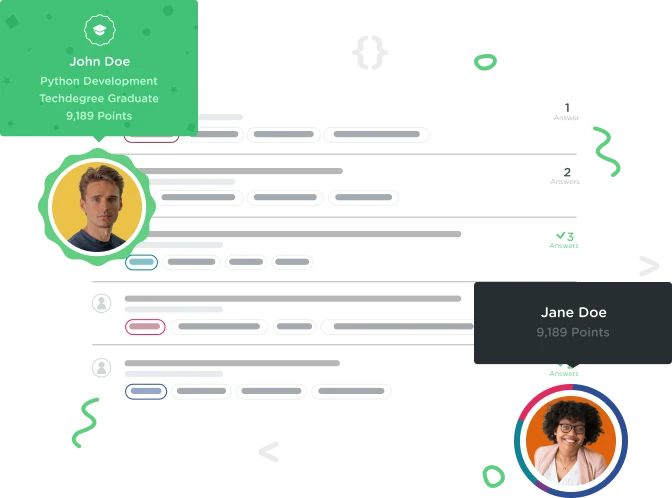

Michael Henry
14,601 PointsExtending random color generation...help please!!!
Hi all,
I am trying to take the random number generation in this exercise one step further by generating a random number for each of the red, blue, and green color channels and combining them to make a completely random, new UIColor each time the button is pressed. What am I doing wrong? Also, how could I make this code more efficient? I feel as if I should not be writing these functions repeatedly.
Thanks for any help. Here is my code...
import Foundation
import UIKit
func redColor () -> Double {
var unsignedArrayCount = UInt32(256)
var unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
var randomRedNumber = Double(unsignedRandomNumber)/255.0
return randomRedNumber
}
func greenColor () -> Double {
var unsignedArrayCount = UInt32(256)
var unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
var randomGreenNumber = Double(unsignedRandomNumber)/255.0
return randomGreenNumber
}
func blueColor () -> Double {
var unsignedArrayCount = UInt32(256)
var unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
var randomBlueNumber = Double(unsignedRandomNumber)/255.0
return randomBlueNumber
}
func randomColor() -> UIColor {
UIColor(red: redColor(), green: greenColor(), blue: blueColor(), alpha: 1.0)
return randomColor()
}
1 Answer

kirkbyo
15,791 PointsHey Michael,
I think I found a solution to your problem. What I decided to do was to create 1 function that will generate one random number as a CGFloat and then created another function that returns a random UIColor.
struct colorWheel {
// Generates a random Random Number
func randomRGB() -> CGFloat {
var unsignedArrayCount = UInt32(255)
var unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
var randomDouble = CGFloat(unsignedRandomNumber)
return randomDouble
}
// Returns a random UIColor
func randomColor() -> UIColor {
let green = randomRGB() / 255.0
let red = randomRGB() / 255.0
let blue = randomRGB() / 255.0
var randomColor = UIColor(red: red, green: green, blue: blue, alpha: 1.0)
return randomColor
}
}
I think the reason why your code was not working was because that UIColor as a double does not accept variables for some reason. That is I decided to use the UIColor that accepts CGFloat.
UIColor(red: CGFloat , green: CGFloat , blue: CGFloat, alpha: CGFloat)
Hope this helped,
Ozzie
Michael Henry
14,601 PointsMichael Henry
14,601 PointsHi Ozzie - I see what you did there, and it makes sense - although shouldn't the red, blue, and green constants be variables so that the random numbers can change each time one clicks the button?
kirkbyo
15,791 Pointskirkbyo
15,791 PointsThe reason why I used
let
was because we are not changing the value of it after we declare it. If you would like to read more about this, I found a helpful answer on Stack Over FlowOzzie
Michael Henry
14,601 PointsMichael Henry
14,601 PointsThanks, Ozzie. Makes sense. Huge help.