Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial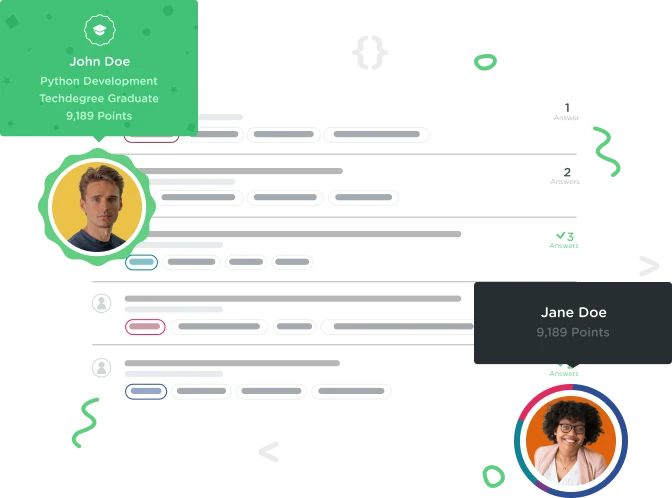
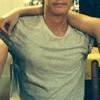
William Hartvedt Skogstad
5,857 PointsExtending the String Type - Swift 2
I can't grasp how one can extend the string type..
Your task is to extend the String type and add a method that lets you add an integer value to the string. For example: "1".add(2) If the operation is possible, return an integer value. If the string does not contain an integer and you cannot add to it, return nil.
// Enter your code below
extension String {
var addInt: Int {
if self.contains(Int) {
return Int?
} else {
return nil
}
}
}
1 Answer
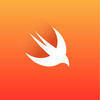
Steven Deutsch
21,046 PointsHey William Hartvedt Skogstad,
You don't want to be using a computed property here, you want to create a method so that you can pass it a value.
We will be extending the String type to give it a method called add(). This method takes in an Int as its only parameter, adds it to the String the method is called on, and returns an optional Int value. The reason why this method returns optional, is because its possible that the String we call the add() method on won't be capable of being used in a math operation. For example, if the String was not composed entirely of number values, and included letters or other special characters.
Therefore, inside the body of this method I used a guard statement to make sure that the String we are calling this method on is capable of being converted to an Int value first. If not, we return nil.
If it was successfully converted to an Int value, we add the value of the number we passed in during the method call and return the resulting value.
// Enter your code below
extension String {
func add(n: Int) -> Int? {
guard let intFromString = Int(self) else {
return nil
}
return intFromString + n
}
}
Good Luck!
William Hartvedt Skogstad
5,857 PointsWilliam Hartvedt Skogstad
5,857 PointsThanks a lot for the complete walkthrough! I was stuck with the mindset from the previous video where the Int type is extended..